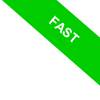
Determining Membership of an Element in a Python Set
In today's Python tutorial, we'll delve into the process of determining whether a particular element is a member of a set. You have two convenient options at your disposal:
The membership operator 'in'
element in set
The contains() method
set.__contains__(element)
Both of these options enable you to verify the presence of an element in a set, which is essentially a set type variable.
It's worth noting that the 'in' operator is often the go-to choice for Python programmers checking for element membership in a set, largely because it's more intuitive and quicker to type compared to its counterpart, the contains() method.
Let's move on to a hands-on example.
Say you're creating a set in a variable, let's call it A, of set type:
>>> A = {2, 4, 6, 8, 10}
You could also employ the set() function to construct the set. Regardless of the method, the outcome is identical.
>>> A = set([2, 4, 6, 8, 10])
Suppose you want to verify the membership of the number 8 in your set.
Using the 'in' operator, you would type '8 in A' on the command line and press enter.
>>> 8 in A
The operator promptly returns True, affirming that the number 8 is indeed a member of the set A.
True
If you were to check the membership of the number 7 in set A in the same way.
>>> 7 in A
You would find the operator returns False, as the number 7 is absent from set A.
True
Here's a practical script example checking for membership using the 'in' operator:
- my_set = {2, 4, 6, 8, 10}
- x = 3
- if x in my_set:
- print("Element 'x' is found in the set.")
- else:
- print("Element 'x' is not found in the set.")
The contains() method also delivers the same results.
For instance, to verify if the number 4 is present in set A, you'd type A.contains(4) in the command line.
>>> A.__contains__(4)
The method returns True, confirming that the number 4 is indeed a member of set A.
True
Using the same method, you can test if the number 3 is in set A.
>>> A.__contains__(3)
In this instance, the method will return False, since the number 3 is not a member of set A.
False
To illustrate, here's a script that checks for element membership using the contains method:
- my_set = {2, 4, 6, 8, 10}
- x = 3
- if my_set.__contains__(x):
- print("Element 'x' is found in the set.")
- else:
- print("Element 'x' is not found in the set.")
Whichever method you choose, you now possess the tools to verify the membership of an element in a set with Python.