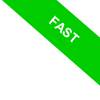
Disjoint Sets in Python
In this comprehensive tutorial, we're going to unpack the concept of determining whether two sets are disjoint using Python.
So, you may be wondering, what are disjoint sets? Essentially, we label two sets as disjoint when they share no common elements. Put simply, if we have two sets, let's say A and B, and they are disjoint, their intersection would be empty, mathematically represented as$$ A \cap B = Ø $$
Python conveniently provides a straightforward method to determine if two sets are disjoint: the isdisjoint() method.
This looks something like:
A.isdisjoint(B)
In this case, A and B are set variables encompassing two distinct sets.
To illustrate this, let's explore a hands-on example.
Let's first establish two sets under the variables A and B.
>>> A = {1, 2, 3, 4}
>>> B = {5, 6, 7, 8}
Now, we'll employ the isdisjoint() method to ascertain whether these sets are disjoint.
>>> A.isdisjoint(B)
The method returns True, indicating that the sets are indeed disjoint as they contain no shared elements.
True
Alternatively, there's another viable approach to validate if two sets are disjoint.
The theory remains the same: two sets are disjoint if their intersection results in an empty set.
$$ A \cap B = Ø $$
Here's a Python code snippet that utilizes this concept to verify if two sets are disjoint, hinging on the cardinality of their intersection.
- A = {1, 2, 3, 4}
- B = {5, 6, 7, 8}
- # Checking disjointness
- if len(A.intersection(B)) == 0:
- print("The sets are disjoint")
- else:
- print("The sets are not disjoint")
This code first sets up two sets within the variables A and B.
Next, the intersection() method is deployed to compute the intersection of the two sets, while the len() method checks if the intersection results in an empty set.
Note that in Python, to calculate the intersection of two sets, we use the intersection() method, and to check the emptiness of a set, we leverage the len() method.
Lastly, an if statement cross-checks if the intersection's length equates to zero. If true, a message is displayed affirming that the two sets are disjoint.
An Alternative Approach
You can also construct the Python code in the following manner:
- A = {1, 2, 3, 4}
- B = {5, 6, 7, 8}
- # Checking disjointness
- if not A.intersection(B):
- print("The sets are disjoint")
- else:
- print("The sets are not disjoint")
This approach brings the 'not' operator into play to determine if the intersection between the two sets results in an empty set, thereby eliminating the need for the len() function to check the intersection's length.
- If the intersection is empty, the 'not' operator returns True and the message "The sets are disjoint" is outputted.
- If the intersection is not empty, the 'not' operator returns False, resulting in the output: "The sets are not disjoint".
All the discussed methods effectively ascertain the disjointness between two sets in Python.