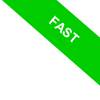
Subsets in Python
This tutorial aims to elucidate how to verify if a given set (A) is a subset of another set (B) in Python. This process leverages the concept of inclusion operators.
Let's dive into a hands-on example.
Firstly, let's create two sets - referred to as A and B - inside the set type variables:
>>> A = {1, 2, 3, 4, 5, 6}
>>> B = {4, 5, 6 }
Now, to check if B is encompassed within A, simply type B<A in your Python terminal.
>>> B<A
For an alternate approach, you could utilize the issubset() method:
B.issubset(A)
Python compares the two sets and provides a Boolean response: True.
True
This indicates that set B is indeed a subset of set A.
Next, test whether set A encompasses set B by typing A>B
A>B
Again, the issuperset() method serves as a valid alternative:
A.issuperset(B)
Here, Python's response is False.
False
Therefore, it's clear that set A does contain subset B.
Next, to check whether set A is encompassed within B, type A<B
A<B
The issubset() method could be utilized again:
A.issubset(B)
Python's response, in this case, is False.
False
Thus, it's established that set A isn't a subset of set B.
For another example, let's create two identical sets.
>>> A = {1, 2, 3, 4, 5, 6}
>>> B = {1, 2, 3, 4, 5, 6}
To check if A is a subset of B, type A<B
>> A<B
The response is False, as the two sets are identical.
False
Hence, A isn't a strict subset of B.
To verify if set A is included in or identical to set B, type A<=B
>> A<=B
Python returns True because every element of A is within B, and the sets are identical.
True
Here, the sets are equal.
To check if set B is included in or identical to set A, type A>=B.
>> A>=B
Python's response is True because all elements of B are contained in A.
True
When two sets are equal A=B, they are referred to as improper subsets of each other.
Let's look at a final example.
Create any set along with an empty set.
A = {1, 2, 3, 4, 5, 6}
B = set()
In this scenario, B is the empty set.
Note. Use the function B=set() to define an empty set in Python. The operator B={ } isn't appropriate as Python interprets the variable B as a dictionary in this case.
Finally, verify if the empty set B is a subset of A.
>>> B<A
Python returns True, acknowledging that an empty set is indeed a subset of any set.
True
The empty set, in this context, is categorized as an improper subset.