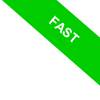
Python's Enumerate Function
In Python the built-in enumerate() function is a handy tool that gives you the ability to add a counter to any iterable object. It’s like having a built-in tally system that keeps track as you loop through an object. Here's the general usage:
enumerate(iterable_object, start_index)
The enumerate() function works with two inputs:
- An Iterable Object
This could be any sequence - be it a string, tuple, and so on, a collection like a set or dictionary, or even an iterator object that you wish to enumerate. - A Starting Index (which is optional)
This represents the initial value that the enumerate() function will use to start its count. If you leave it unspecified, the 'start_index' is set to zero by default.
In essence, what the enumerate() function does is it counts the elements as it iterates, providing a tuple - that is, a pair of values, comprising the iterable's element and the running count.
Allow me to illustrate with a practical example.
Suppose you have a list (or any other iterable object)
>>> fruits = ['apple', 'banana', 'mango', 'grape', 'orange']
Now, you want to transform this list into an enumerable iterator object. The enumerate() function comes into play.
>>> obj=enumerate(fruits)
The function will yield an iterator object of the class "enumerate".
Don't just take my word for it - you can confirm this by using the type() function.
>>> type(obj)
<class 'enumerate'>
From this point, you can iterate through the elements, from the first to the last, using the next() function.
next(obj)
Python will promptly serve up a pair of values - the count and the corresponding element from your list.
To start with, the counter is zero.
(1, 'banana')
As you keep calling the next() function, Python dutifully displays the subsequent tuples:
>>> next(obj)
(1, 'banana')
>>> next(obj)
(2, 'mango')
>>> next(obj)
(3, 'grape')
>>> next(obj)
(4, 'orange')
After the last element has been served, any subsequent call to next() will result in Python raising a StopIteration exception.
Besides the next() function, you can also peruse the content of the enumerate object using a for loop.
- for i, fruit in enumerate(fruits):
- print(f"Elemento {i}: {fruit}")
Running this loop will yield:
Elemento 0: apple
Elemento 1: banana
Elemento 2: mango
Elemento 3: grape
Elemento 4: orange
Here, "i" represents the running count while "fruit" stands for the value of the element.
That's Python's enumerate() function for you - neat, elegant, and powerful.