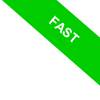
Union of Two Sets in Python
In this tutorial, we'll explore set operations in Python, with a specific focus on the union operation.
Let's learn through a practical example.
Kick things off by creating two sets. We'll use the set() function and assign them to variables 'A' and 'B'.
>>> A=set([1,2,3,4,5])
>>> B=set([4,5,6,7])
Another way to achieve the same is by defining the sets using curly braces, bypassing the set() function entirely.
>>> A={1,2,3,4,5}
>>> B={4,5,6,7}
Now, when it comes to finding the union of two sets, we have two main avenues:
The Union Operator: A|B
The '|' operator allows us to merge the two sets seamlessly.
A|B
We execute the command C=A|B
>>> C=A|B
What we get is the union set A∪B
>>> C
{1, 2, 3, 4, 5, 6, 7}
This union set comprises all elements that are part of set A and/or set B, excluding any duplicates.
The Union() Method
Another approach to deriving the union is using the .union() method.
A.union(B)
Run the command A.union(B)
>>> D=A.union(B)
Alternatively, it's possible to use set.union(A,B). The outcome remains the same.
>>> D=set.union(A,B)
This yields the union set D=A∪B
>>> D
{1, 2, 3, 4, 5, 6, 7}
The union set D contains every element from sets A and B, again, with no duplications.
It's worth noting the distinction between the '|' operator and the union() method. Both are effective methods to obtain the union of two sets. However, the .union() method carries an added flexibility as it can also handle iterable objects in addition to sets. For instance, let's create a set A and a list E:
>>> A=set([1,2,3,4,5])
>>> E=[4,5,6,7]
Applying the .union() method on set A with list E gives us a union, which is a set.
>>> A.union(E)
{1, 2, 3, 4, 5, 6, 7}
Conversely, attempting to use the union operator A|E will result in a Python error. This is because the '|' operator strictly operates between set variables.
>>> A|E
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: unsupported operand type(s) for |: 'set' and 'list'
The union() method also facilitates merging multiple sets at once.
For example, let's define three sets A, B, and C.
>>> A=set([1,2,3,4,5])
>>> B=set([4,5,6,7])
>>> C=set([7,8,9])
We then unite these three sets using the union() method, listing the sets as arguments.
>>> D=A.union(B,C)
This provides us the union set D=A∪B∪C
>>> D
{1, 2, 3, 4, 5, 6, 7, 8, 9}
By now, you should have a comprehensive understanding of how to compute the union of two or more sets in Python.