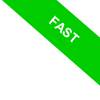
Python's iter() Function
Peek into the world of Python, and you'll soon encounter the nifty iter() function. This handy tool allows you to transform a regular object into an iterator.
iter(x)
Simply call iter(x), with 'x' being the object you wish to make iterable. This could be an array of items like a list, dictionary, or string, you name it.
With your object now iterable, you've unlocked the ability to sift through its elements, one after the other, in sequence.
Now, you might ask, "What's this iterator you speak of?" Well, it's an object that allows you to traverse through it, serving up one element at a time. To pull these elements, you could enlist the help of a for loop, the __next__() method, or the next() function.
Let's paint a clearer picture with a practical example.
Imagine you create a list.
>>> my_list = [1, 2, 3, 4, 5]
The list is an iterable object but doesn't quite cut it as an iterator yet, as it's missing the __next__() attribute.
>>> hasattr(my_list, '__next__')
False
You can grant it the status of an iterator using the iter() function.
>>> obj = iter(my_list)
Or, if you prefer, you could call upon the list's __iter__() method to achieve the same effect.
>>> obj = my_list.__iter__()
The object we've named "obj" is now in the elite club of iterators, possessing the __next__() method.
>>> hasattr(obj, '__next__')
True
This means you can freely pluck its elements, sequentially from the first to the last, utilizing the next() function.
>>> next(obj)
1
>>> next(obj)
2
>>> next(obj)
3
>>> next(obj)
4
>>> next(obj)
5
>>> next(obj)
Traceback (most recent call last):
StopIteration
When you've exhausted all elements to iterate over, the next() method triggers the "StopIteration" exception.
In some scenarios, Python does the heavy lifting for you, automatically converting an iterable object into an iterator, no manual intervention required.
For instance, consider when you're using a for loop:
- my_list = [1, 2, 3, 4, 5]
- for item in my_list:
- print(item)
In this situation, the for loop automatically calls upon the iter() function, crafting an iterator and proceeding to read an element at each iteration.
Here's how the output of this script would look:
1
2
3
4
5
And here's the cherry on top: the for loop also takes care of handling the StopIteration exception behind the scenes.
As such, you won't see the exception rearing its head on your screen. Neat, isn't it?