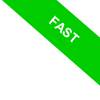
Set Difference in Python
In this Python tutorial we'll delve into the concept of set difference.
We'll be exploring different ways to calculate the difference between two or more sets in Python, each with its unique uses and properties.
The difference operator A-B
Let's start with a basic operator: A-B.
This operator provides a quick way to determine the difference between two sets.
A-B
To give you a clear example, let's create two sets, A and B, using the set() function.
>>> A=set([1,2,3,4,5])
>>> B=set([4,5,6,7])
Then, by simply typing C=A-B in the command line, we can find the difference.
>>> C=A-B
The result will be the set difference A-B.
>>> C
{1, 2, 3}
Essentially, the set difference gives us the elements in set A that don't exist in set B. In our example, these are elements 1, 2, and 3.
The difference() method
Now let's move to a slightly different approach: the .difference() method.
A.difference(B)
With the command A.difference(B), we can achieve the same outcome.
Here is another practical example for clarity.
Create two sets, A and B,
>>> A=set([1,2,3,4,5])
>>> B=set([4,5,6,7])
Then, type A.difference(B) into the command line,
>>> D=A.difference(B)
Alternatively, you can use the syntax set.difference(A,B) to achieve an identical result.
>>> D=set.difference(A,B)
And voila! The result is the same set difference A-B.
>>> D
{1, 2, 3}
The set difference D is made up of elements from set A that don't also belong to set B.
In this case, these are the elements 1, 2, and 3.
What separates the A-B operator from the difference(A,B) method? Well, while they both yield the same result, the .difference() method offers a bit more flexibility. For instance, it works not only with sets but also with other iterable objects like lists or tuples. Let's create a set variable A and a list E to demonstrate:
>>> A=set([1,2,3,4,5])
>>> E=[4,5,6,7]
The .difference() method works perfectly, giving you the difference between the set (A) and the list (E).
>>> A.difference(E)
{1, 2, 3}
However, the A-B operator wouldn't work in this scenario. Attempting to run A-E would return an error because Python only allows the '-' operator between sets.
>>> A-E
Traceback (most recent call last):
TypeError: unsupported operand type(s) for -: 'set' and 'list'
The .difference() method also shines when dealing with multiple sets in sequence.
Imagine you have three sets A, B, and C
>>> A=set([1,2,3,4,5])
>>> B=set([4,5,6,7])
>>> C=set([2,4,6,8])
You can calculate the difference in one go.
>>> D=A.difference(B,C)
The final result is the set difference D=(A-B)-C, which contains elements 1 and 3.
>>> D
{1, 3}
The difference_update() method
Lastly, let's talk about the difference_update() method. It's unique because it calculates the set difference and modifies the original set in the process.
A.difference_update(B)
Here's an example for better understanding.
Create two sets A and B.
>>> A=set([1,2,3,4,5])
>>> B=set([4,5,6,7])
Type A.difference_update(B) to compute the difference,
>>> A.difference_update(B)
And you end up with the set difference directly in A, altering its content.
>>> A
{1, 2, 3}
In this case, the outcome has directly altered the content of set A.
So what separates the difference and difference_update methods? Essentially, both methods find the difference between two sets. However, the difference method leaves the original sets untouched, while the difference_update method alters the original set it is applied on.
That's all for this tutorial. I hope you've found it helpful in understanding how to compute set differences in Python, each method with its own unique capabilities. Happy coding!