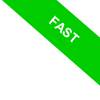
Python's len() Function
The len() function in Python is a versatile tool for determining the length or count of elements in iterable objects such as strings, lists, tuples, among others.
len(obj)
"obj" in this context refers to the iterable object whose length you're interested in determining.
This function is straightforward: it returns the total number of elements in an iterable object.
Note: When applied to an empty object, len() returns 0. It's also important to remember that len() isn't compatible with non-iterable data types, like integers or floating-point numbers. Attempting to do so will trigger a TypeError in Python.
How len() behaves and counts elements depends on the specific iterable in question.
This guide includes practical examples for each type of iterable, giving you a hands-on understanding of len()'s versatility.
Strings and len()
Discover the length of a string—essentially the number of characters it contains—using len().
Consider this string example:
string = "Hello World"
Passing this string to len() like so:
print(len(string))
will yield the character count of the string. Here, it's 10.
10
Keep in mind, spaces are counted as characters and are included in the total.
len() with Lists
len() is equally effective for counting the elements in a list.
Take this list as an example:
list = [1, 2, 3, 4, 5]
To find the number of items in the list:
print(len(list))
The list contains five elements.
5
What about an empty list?
An empty list will return a length of zero.
For example:
empty_list = []
Counting its elements with len() results in:
print(len(empty_list))
which is zero.
0
Tallying Elements in Tuples with len()
len() also serves to determine the size of tuples, functioning similarly as with lists.
Consider this tuple:
tuple = (1, 2, 3)
Using len() here:
print(len(tuple))
reveals that the tuple has three elements.
3
len() and Dictionaries
When applied to dictionaries, len() indicates the number of key-value pairs present.
For instance, here's a dictionary:
dictionary = {'a': 1, 'b': 2, 'c': 3}
Using len() to count its key-value pairs:
print(len(dictionary))
shows there are three pairs.
3
Working with Sets and len()
The len() function is also applicable to sets, where it counts the unique elements.
Here's a set with five elements:
set = {1, 2, 3, 4, 5}
Using len() to count these elements:
print(len(set))
confirms there are five unique elements.
5
As sets hold only unique elements, len() will always return the count of distinct items. Duplicates, if any, are removed automatically.
Consider this set with duplicates:
set_with_duplicates = {1, 2, 2, 3, 3, 3, 4, 4, 4, 4}
Counting its elements:
print(len(set_with_duplicates))
reveals only four unique values, despite being defined with ten.
4
By now, you should have a comprehensive grasp of the len() function. It's an invaluable tool in Python, essential for a variety of programming tasks.