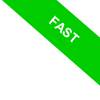
Immutable Sets (frozenset) in Python
In this tutorial, we will explore how to create an immutable set in Python.
So, what exactly is an immutable set? An immutable set or a constant set, as the name suggests, is a collection of elements that cannot be altered. That is, you can neither add new elements to the set nor remove any existing ones. For instance, an empty set is immutable because it is impossible to add or remove elements from it.
In the Python language, this immutable quality is achieved by employing the 'frozenset' function, wherein 'x' represents a collection of elements:
frozenset(x)
Here, x refers to a collection of elements.
Moving forward, let's delve into a practical application of this function.
We will create an immutable set, which we will store in variable A:
>>> A=frozenset([2,4,8,10])
Once A takes form, it becomes unalterable.
Any attempt to add new elements or eliminate existing ones will be met with a stern response from Python.
>>> A.add(12)
AttributeError: 'frozenset' object has no attribute 'add'
Nevertheless, this immutability doesn't limit a frozenset's involvement in union, intersection, and difference operations with the conventional sets.
To exemplify, let's create two sets, A and B.
>>> A=frozenset([2,4,8,10])
>>> B=set([1,3,5,7,9])
Following this, we will merge A and B using the union operator A|B
>>> C=A|B
This operation results in a new set, C, which is of the frozenset type, signifying its immutability.
>>>C
frozenset({1, 2, 3, 4, 5, 7, 8, 9, 10})
To confirm C's type.
>>> type(C)
<class 'frozenset'>
To ascertain if two frozensets are disjoint, methods such as isdisjoint(), issubset(), and issuperset() come in handy.
For instance, consider two frozenset sets, A and B.
>>> A=frozenset([1,2,3,4,5])
>>> B=frozenset([2,3])
A.isdisjoint(B) will allow us to determine if the sets are disjoint.
In this particular case, they are not.
>>> A.isdisjoint(B)
False
What's worth noting is that frozenset objects can reside within a mutable set.
For instance, let's have two immutable sets (frozensets) within variables A and B.
>>> A=frozenset([2,4,8,10])
>>> B=frozenset([1,3,5,7,9])
Now, we'll create a mutable set, C, which contains the frozensets A and B.
>>> C=set([A,B])
Python handles this gracefully without throwing any errors.
The set C now nests the two frozensets.
{frozenset({1, 3, 5, 7, 9}), frozenset({8, 2, 10, 4})}
This demonstrates an interesting aspect of sets: while a standard set cannot include other sets within it, frozensets are an exception to this rule.