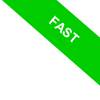
Symmetric Difference Between Sets in Python
How do we find the symmetric difference between two sets in Python? In this guide, we will explore this in an easy manner.
Firstly, we need to understand what symmetric difference is. Simply put, it represents the elements that belong to either set but not both. $$ (A - B) ∪ (B - A) $$ For example, let's consider two sets $$ A = \{ 1, 2, 3 \} $$ $$ B = \{ 2, 3, 4 \} $$ Their symmetric difference, (A - B) ∪ (B - A), would then be {1, 4}. $$ (A - B) ∪ (B - A) = \{ 1 \} ∪ \{ 4 \} = \{ 1, 4 \} $$.
Now, let's see how we can compute this in Python. There are two primary ways:
Using the symmetric difference operator (^)
You can use the caret (^) operator to calculate the symmetric difference between two sets.
A^B
Here's a quick example:
First, create two sets in variables A and B using the set() function.
>>> A=set([1,2,3,4,5])
>>> B=set([4,5,6,7])
Now type C=A^B in your Python terminal.
>>> C=A^B
The result is the symmetric difference of the two sets.
>>> C
{1, 2, 3. 6. 7}
The symmetric difference set contains elements that are found in one of the two sets but not in both.
$$ (A - B) ∪ (B - A) = \{ 1,2,3 \} ∪ \{ 6,7 \} = \{ 1, 2,3,6,7 \} $$
In this case, these are the elements 1, 2, 3, 6, and 7.
The symmetric_difference() Method
You can also obtain the symmetric difference between two sets using the .symmetric_difference() method of the set variables.
A.symmetric_difference(B)
Let's see how it works.
Create two sets A and B.
>>> A=set([1,2,3,4,5])
>>> B=set([4,5,6,7])
Assign the result of the operation A.symmetric_difference(B) to a variable D.
>>> D=A.symmetric_difference(B)
Alternatively, you could write D=set.symmetric_difference(A,B). The result is the same.
>>> D=set.symmetric_difference(A,B)
The result is the symmetric difference of the two sets.
>>> D
{1, 2, 3, 6, 7}
The symmetric difference set (D) comprises elements that are found in the first set (A) but not in the second (B) and elements that are found in the second set (B) but not in the first (A).
$$ (A - B) ∪ (B - A) = \{ 1,2,3 \} ∪ \{ 6,7 \} = \{ 1, 2,3,6,7 \} $$
In this case, these are the elements 1, 2, 3, 6, and 7.
What's the difference between the A^B operator and the symmetric_difference(A,B) method? Both methods allow you to calculate the symmetric difference between two sets. However, the .symmetric_difference() method is more versatile. For instance, you can use the symmetric_difference() method with set variables and other iterable objects (lists, tuples, etc.). For example, create a set variable A and a list E.
>>> A=set([1,2,3,4,5])
>>> E=[4,5,6,7]
The .symmetric_difference() method processes and returns the symmetric difference between the set (A) and the list (E), even if they are objects with different structures. The result is a set.
>>> A.symmetric_difference(E)
{1, 2, 3, 6, 7}
On the other hand, the ^ operator can only be used between set variables. If you try to process the command A^E, Python returns an error because the variable E is a list.
>>> A^E
Traceback (most recent call last):
TypeError: unsupported operand type(s) for ^: 'set' and 'list'
Also, unlike other set variable methods, the symmetric_difference() method allows you to work only between two sets. If you use the symmetric_difference() method with three or more sets, Python returns an error. For example, define three sets A, B, and C.
>>> A=set([1,2,3,4,5])
>>> B=set([4,5,6,7])
>>> C=set([2,4,6,8])
Then, calculate the symmetric difference using the symmetric_difference() method.
>>> D=A.symmetric_difference(B,C)
In this case, Python returns an error message.
D=A.symmetric_difference(B,C)
TypeError: set.symmetric_difference() takes exactly one argument (2 given)
D=( (A-B)&(A-C) ) | ( (B-A)&(B-C) ) | ( (C-A)&(C-B) )
The result is a set with elements that belong to only one of the sets A, B, and C but not to the others. In this case, these are the elements 1, 3, 7, and 8.>>D
{1, 3, 7, 8 }
The symmetric_difference_update() Method
The symmetric_difference_update() method calculates the symmetric difference between two sets by modifying the set it is applied to.
A.symmetric_difference_update(B)
Let's look at a practical example.
Create two sets A, B:
>>> A=set([1,2,3,4,5])
>>> B=set([4,5,6,7])
Type A.symmetric_difference(B) on the Python command line:
>>> A.symmetric_difference_update(B)
The result is the set of the symmetric difference A=(A-B)U(B-A), which includes these elements:
>>> A
{1, 2, 3, 6, 7}
In this case, the result modifies the content of the set A.
So, what's the difference between the symmetric_difference and symmetric_difference_update methods? Both calculate the symmetric difference between two sets. However, symmetric_difference_update alters the content of the first set, whereas symmetric_difference creates a new set without altering the other sets involved in the operation.