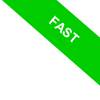
Flush() Method in Python
The flush() method in Python is a crucial tool for managing the buffer during file write operations. It allows you to clear the buffer and immediately commit your data to a file.
file.flush()
Essentially, this method ensures the direct transfer of data from the memory buffer to the target file, bypassing the need for the buffer to fill up first. It's particularly valuable for writing files or sending data across networks, enhancing the reliability and timeliness of data transfer.
Purpose: Python optimizes data handling by temporarily storing it in a buffer before writing to a file. This process typically waits until the buffer is full to "flush" the data to its destination. The flush() method empowers you to initiate this transfer on-demand, mitigating the risk of data loss during unexpected interruptions or errors.
Here's a real-world example for clarity.
with open('log.txt', 'w') as file:
file.write('Log start\n')
file.flush()
# Imagine this space filled with critical operations
file.write('Important action completed\n')
file.flush()
In this scenario, regardless of the number of operations between writings, the flush method guarantees that messages are promptly recorded in the log file immediately after they are generated. This approach is invaluable for ensuring immediate data documentation or transmission.
Especially in scenarios requiring real-time interaction or concurrent execution of multiple programs (such as multithreading or multiprocessing), the ability to send every piece of information instantly is paramount.
Yet, it's wise to note that overusing flush can impact program efficiency by circumventing the designed buffering system that optimizes I/O operations.
Therefore, strategic use is recommended to balance immediate data needs with overall program performance.