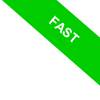
Python's read() Method
The read() method in Python is a powerful tool that enables you to load a file’s contents into your computer’s memory, offering the flexibility to process it according to your needs.
file.read()
When applied, this method efficiently reads the entirety of a file opened in 'read' mode ('r'), delivering the content as a single string.
Upon reaching the file's end, the read() method returns an empty string.
By default, the read operation treats files as text, presenting the data as string type.
Binary files are no exception to the read() method's capabilities. Whether you're dealing with files in 'rb' mode (binary read), the read() method functions seamlessly, returning the data in byte string format without any additional steps required.
Here’s a practical example for clarity.
To begin reading a file, it’s essential to first open it using the open() function in 'r' mode for reading.
file = open('example.txt', 'r')
With the file open, invoking the read() method allows you to access its full content.
content = file.read()
Executing the method without any parameters ensures the entire file is read from start to finish.
This content is then stored as a text string, ready for further manipulation, such as displaying it on the screen with a print() statement,
print(content)
It’s considered best practice to close the file with the close() method once you are finished, to free up resources.
file.close()
Python also provides a more elegant solution for managing file closures using the with statement. This approach ensures files are automatically closed after their block of code has executed, removing the need for manual closure.
with open('example.txt', 'r') as file:
content = file.read()
print(content)
Reading File Portions Instead of the Whole
For large files, it may be more efficient to read only a specific portion to conserve memory.
The read() method can be fine-tuned by passing a numerical argument that specifies the number of bytes to be read, such as read(100) to read the first 100 characters (or bytes).
file = open('example.txt', 'r')
partial_content = file.read(100)
print(content)
file.close()
To continue reading in increments of 100 characters, simply invoke the read() method again.
file = open('example.txt', 'r')
partial_content = file.read(100)
print(content)
partial_content = file.read(100)
print(content)
file.close()
Line-by-Line Reading
Reading a file line by line is especially useful for text files comprised of multiple lines or records.
The readline() method is designed for this purpose, allowing for the sequential reading of individual lines.
file = open('example.txt', 'r')
first_line = file.readline()
print(first_line)
file.close()
To read several lines, repeat the readline() command as needed.
For instance, to access the first two lines, your code might look like this:
file = open('example.txt', 'r')
# reading the first line
first_line = file.readline()
print(first_line)
# and now the second
second_line = file.readline()
print(second_line)
file.close()
For a comprehensive view, the readlines() method returns a list of all lines, enabling bulk reading and individual line processing.
file = open('example.txt', 'r')
all_lines = file.readlines()
print(all_lines)
file.close()
This method simplifies access to the entire file, allowing for targeted access to records of interest without the need for line-by-line reading.
To illustrate, accessing the last record of a file is straightforward:
with open('diary.txt', 'r') as file:
lines = file.readlines()
last_line = lines[-1]
print("Latest diary entry:", last_line)
This method focuses on the last line, showcasing the most recent entry in your diary.