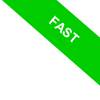
Python's write() Method
In Python, the write() method serves the essential function of writing a string to a file.
write(string)
You need to specify the string you wish to write to the file within the parentheses.
Keep in mind, to leverage write(), you must first open the file using the open() function in either write ('w') or append ('a') mode.
- 'w' opens the file for writing, erasing any existing content
- 'a' appends to the end of the file, preserving existing content.
The write() method doesn't directly commit data to the file; instead, it's buffered in memory to enhance performance. To ensure all data gets written, it's crucial to flush this buffer by either calling flush() or closing the file with the close() method at the end of your operation.
Let's look at a practical example to illustrate creating a file, writing text to it, and then closing it:
First, open the file in write mode.
file = open('example.txt', 'w')
With the file opened, the write() method allows you to write a string to it.
file.write('Hello, world!\n')
While the file is in write mode, you can execute multiple write operations.
Each write() invocation appends text to the file.
file.write('Hello, world!\n')
file.write('Welcome to the exciting world of Python.\n')
In this scenario, two lines of text have been added to "example.txt".
Observe the \n symbol at each string's end, crucial for starting a new line in the file. Without it, all text would be on a single line.
Closing the file with the close() method when finished is recommended for good practice.
file.close()
This ensures the memory buffer is fully written to the file and frees up the resources engaged during writing.
For a streamlined approach to file handling, consider using the with statement.
with open('example.txt', 'w') as file:
file.write('Hello, world with with!\n')
file.write('This method automatically takes care of closing the file.\n')
The with statement simplifies file management by automatically closing the file, eliminating the need for a manual close() call.
This approach is not only tidier but also safer, ensuring files are properly closed even in the event of a writing error.
Appending Text to an Existing File
To add content to an existing file without overwriting it, open the file in 'a' (append) mode.
file = open('example.txt', 'a')
file.write('Adding a new line to the existing file.\n')
file.close()
This method allows for the addition of a new line to "example.txt", seamlessly integrating with the existing content.