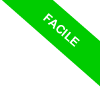
Python's readline() Method Explained
The readline() method in Python is a straightforward yet powerful tool for reading text files line by line. This approach is particularly beneficial for processing large files or analyzing content on a record-by-record basis without loading the entire file into memory.
file.readline()
Imagine you're working with a text file named `poetry.txt`, containing a poem with each verse starting on a new line.
Here’s an effective way to use readline to echo every verse to the console:
with open('poetry.txt', 'r') as file:
while True:
# Read a line
line = file.readline()
# An empty line indicates the end of the file
if not line:
break
# Output the line, newline character included
print(line, end='')
Start by opening the file with the open() function in read mode (`'r'`).
Next, employ the readline() method to sequentially read a line at a time. With each invocation, Python fetches the subsequent line from the file.
The print(line, end='') command displays each line without appending an additional newline character, leveraging the fact that readline() already includes a newline character at the end of each line it reads, except for the file's last line if it doesn't end with a newline.
Upon reaching the file's end, readline() returns an empty string (''), serving as a cue to break out of the loop reading the file.
The perpetual while True: loop is cleverly terminated using a break command once readline() signals the file's conclusion with an empty string.
To wrap up, closing the file with the close() method is a best practice, ensuring the release of system resources. However, in this script, the use of a context manager (with open(...) as file:) obviates the need for explicitly closing the file, as it's automatically handled at the end of the block.