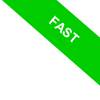
Handling Files in Python
Today, we're delving into Python's approach to file handling.
But first, what exactly are files? Files are essentially digital receptacles, perfect for storing varied forms of information, be it text, images, or sounds. They differ from memory-stored variables in one key aspect - they are saved to your computer's hard drive. This ensures that the data written to them remains accessible, even post-reboot.
In Python, files are dealt with as objects. These objects permit operations such as reading, writing, and editing. Let's begin to unravel how this works.
Opening a File
The process of opening a file in Python involves the open() function, which provides a file object.
This object serves as your interface with an actual file residing in your computer's file system.
Here's a practical example.
file = open("test.txt")
Here, the file is opened in read mode, which, by default, is Python's chosen mode.
This file object presents various attributes, like the file name and character encoding, as well as several methods for reading, writing, or editing its content.
Among these methods, read(), readlines(), write(), and close() are crucial.
Writing to a File
Writing data into a file involves opening the file in 'write' mode. The 'w' (write mode) serves as the second argument in the open() function.
This illustrates it:
file = open("test.txt", 'w')
Once opened, you can write records into the file using write()
file.write("Hello world!")
This action adds the string "Hello world!" to the file.
You may repeat the write() operation for every record you wish to add.
Each record ends with an automatically appended newline character \n. This control character signals Python that the record has ended.
Once your write operations are done, it's good practice to close the file using close().
file.close()
The close() operation clears the file object from memory and releases the actual file from write operations.
Appending Records to an Existing File. The 'w' write mode is useful for creating new files. However, if you use it to write records to an existing file, Python will overwrite the previous contents. Should you need to append records to an existing file without overwriting the current content, use 'append' mode, denoted by "a" as the second argument in the open() method.
file = open("test.txt", 'a')
file.write("Hello world!")
file.close()
In this case, Python adds the new record to the end of the file without overwriting existing records.
Reading a File
Reading a file's content can be done using read().
The file first needs to be opened in 'read' mode, marked by "r" as the second argument in the open() method.
file = open("test.txt", 'r')
Alternatively you can also omit the second argument, as the open() method defaults to read mode.
file = open("test.txt")
With the file opened in read mode, you can now use read() to obtain the content of each record.
record = file.read()
This last statement reads a record's content and assigns it to the "record" variable.
Repeat the read() method to read the remaining records, or use a for loop to read all the file's lines.
- for line in file:
- print(line, end='')
Another useful method is readlines(), which returns a list of all the file's records.
lines = file.readlines()
This method returns a list of all the file's records in one operation.
Upon reaching the file's end, the read() method returns an empty string.
Ensure to close the file with the close() method once you're done with reading operations.
file.close()
Closing a File
Closing files is a vital step. It can be done using the close() method.
This is done using the close() method.
- file = open("test.txt")
- print(file.read())
- file.close()
Alternatively, the with keyword can be used, which ensures the file is automatically closed at the end of the code block, providing cleaner code and handling errors effectively.
- with open("test.txt") as file:
- print(file.read())
In this instance, the close() method is not necessary because Python automatically takes care of it.
The code block following the with keyword must be indented appropriately, as Python relies on indentation to identify code blocks.
Remember to indent the code block to the right of the with keyword, as Python uses indentation to identify code blocks.
These rudimentary concepts underpin Python's file handling mechanism.
More advanced operations, like handling binary files, will be covered in subsequent lessons.
Getting to Know the open() Function Parameters
The open() function is equipped with several parameters that are essential for handling files efficiently. Let’s dive into a thorough explanation of its primary parameters:
- file: Specifies the path to the file you wish to open. This can be a string that points to either an absolute or relative path or an object that adheres to the `os.PathLike` interface, which then returns a path.
- mode: Defines how the file will be opened. Here are the modes you’re likely to use:
- 'r': opens the file for reading only (this is the default setting).
- 'w': opens the file for writing, replacing it if it exists or creating a new one if it doesn’t.
- 'x': exclusively opens the file for writing. If the file already exists, the operation fails.
- 'a': opens the file to append data at its end if it exists or creates a new one if it doesn’t.
- 'b': opens the file in binary mode, where the output is a byte string. This can be combined with other modes like 'rb', 'wb', 'xb', etc.
- 't': opens the file in text mode (this is the default), meaning the output and input will be strings.
- '+': opens the file for both reading and writing, applicable in several modes like 'r+', 'w+', 'x+', 'a+'.
Example:f = open('myfile', 'w')
- buffering: Specifies the buffer size for file operations. A `0` value means no buffering, applicable only in binary mode, `1` is for line buffering, automatically flushing the buffer with each new line (only in text mode), and larger numbers define the buffer size in bytes. Example:
f = open('myfile', 'w', buffering=1)
- encoding: Sets the encoding for the file’s content. If omitted, Python defaults to the system’s encoding. Example:
f = open('myfile', 'w', encoding='latin1')
- errors: Offers options for managing encoding and decoding errors. Choices include 'strict' for throwing exceptions, 'ignore' to overlook errors, and 'replace' to substitute problematic characters. Example:
f = open('myfile', 'w', errors='ignore')
- newline
Manages how new lines are treated. The default 'None' converts all new lines to '\n', while other settings allow for no conversion or specify different new line characters. Example:f = open('myfile', 'w', newline='\r')
- closefd: When opening a file with a file descriptor rather than a name, set this to `False` to prevent the descriptor from closing once the file object does. The default `True` closes the file along with the file object.
- opener: Allows for a custom opener function that takes `(file, flags)` and returns an opened file descriptor, useful for customizing file opening procedures.