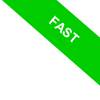
Serialization in Python
Welcome to this tutorial where we'll demystify the concept of serialization in Python. While it may initially appear daunting, it becomes quite straightforward with a few hands-on examples.
What exactly is serialization? It's a crucial technique in programming that allows you to store an object or data for later use, either in a different session or in a subsequent run of your program. Imagine it as a method for 'freezing' and later 'thawing' objects, transforming them into a format that's effortlessly storable and retrievable. A classic example is saving your progress in a video game to resume at a later time – all made possible through serialization.
Serialization is incredibly useful when you need to maintain an object's state after your program has ended, or if you're sending the object over a network.
Understanding Python's Pickling
Python refers to serialization as "pickling".
This process is similar to preserving food in a jar – you're essentially 'jarring' your objects in a virtual space, commonly a file on your computer, using Python's pickle module.
Let's dive into a practical example for clarity.
Begin by importing the pickle module.
import pickle
Create an object, such as a dictionary with some data.
my_dictionary = {"name": "Mario", "age": 30}
Then, you open a file using the pickle method with the 'wb' parameter to write the object.
Follow this by saving the object using the pickle.dump() method.
- # Writing data to file
- with open('my_dictionary.pkl', 'wb') as file:
- pickle.dump(my_dictionary, file)
Your dictionary data is now securely stored in the file "my_dictionary.pkl" on your PC, accessible from the Python directory.
For retrieval, the deserialization process comes into play.
Deserialization: Bringing Data Back to Life
To reuse your serialized object, turn to "unpickling". This is akin to reopening the jar and returning its contents to their original form.
For instance, to retrieve your dictionary, open the "my_dictionary.pkl" file with the pickle method using the 'rb' parameter.
Load the data back into memory with the pickle.load() method.
- # Retrieving data from file
- with open('my_dictionary.pkl', 'rb') as file:
- my_loaded_dictionary = pickle.load(file)
Finally, display the contents of your loaded variable.
print(my_loaded_dictionary)
The screen will now show the data you had initially stored.
In this example, the original dictionary is presented:
{'name': 'Mario', 'age': 30}
A crucial note of caution: always be wary of using serialized data from unknown or untrusted sources. The process of unpickling can potentially execute harmful code.
The Shelve Module
If you're looking for an alternative method to serialize objects, consider the Shelve module.
Begin by importing the module:
import shelve
To write serialized data, use the shelve.open() method. Here's how:
- # Creating and storing data in the shelve
- shelf = shelve.open('test')
- shelf['name'] = 'Mario'
- shelf['age'] = 30
- shelf.close()
To access your stored data, simply employ the same shelve.open() method.
For example, start a new Python session to reset the memory, and then reload your serialized data:
- # Opening the shelve and accessing the data
- shelf = shelve.open('test')
- print(shelf['name'])
- # Remember to close the shelve when done
- shelf.close()
This process allows Python to retrieve and display the value linked to the "name" key, in this case, "Mario".
Mario
This approach is an efficient way to maintain and access data over time, offering a practical solution for data serialization in Python.