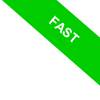
Python's close() Method
The close() method in Python is essential for properly terminating a network connection or closing a file once you're done with it.
file.close()
Its primary function is to conclude a session with a resource, such as a file, ensuring that any allocated resources are released. This step is vital to prevent memory leaks or execution errors due to unreleased resources.
Why is it crucial? Consider the analogy of borrowing a book from a library: you take it off the shelf, read it, but then leave it open on a table without putting it back. Eventually, the library would become a chaotic mess, making it impossible to find anything. Similarly, in the digital domain of your computer's memory, files act as the books that need proper management to avoid disarray.
Here's a concrete example for clarity.
Imagine you're jotting down some notes in a file named "my_notes.txt".
First off, you open the file in write mode with the open() function.
file = open('my_notes.txt', 'w')
Next, you add your notes to the file using the write() method.
file.write("Today I learned something new about Python!")
Once you're done, it's imperative to close the file with the close() method to ensure all your changes are saved and the resources are freed for future use.
file.close()
This action is akin to returning a book to its rightful place after reading, keeping everything organized and accessible.
No Need for close() in a with Block
If remembering to close files is a challenge, the with statement offers a more foolproof solution. It automatically manages the closure of resources, making your code cleaner and more robust.
For instance, the same task mentioned above can be accomplished with the following syntax:
with open('my_notes.txt', 'w') as file:
file.write("Today I learned something new about Python!")
This method ensures the file is automatically closed once the block is exited, removing the need for a separate close() call. It's a safer, more efficient way to handle files, especially when errors might occur during processing.