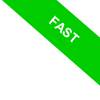
The writelines() Method in Python
In this tutorial, we delve into the writelines function in Python, a pivotal tool for file manipulation. This function excels when you're tasked with writing a list of strings to a file, streamlining the process significantly.
writelines(data)
Writelines takes "data" as its argument within parentheses—an iterable object made up of strings—and efficiently writes them to a file.
Setting it apart from the write function, which processes a single string at a time, writelines empowers you to elegantly dispatch an entire list of strings to your file in one fell swoop. This approach is not only more convenient but also enhances productivity when handling multiple lines.
A pro tip: ensure you include newline characters "\n" within your strings to have them appear on separate lines in your file. Neglecting this will result in a continuous string written across a single line.
Let's start with a simple example to set the stage.
Create a list, essentially an iterable object, brimming with strings.
lines = ["Hello world!\n", "Here's an example of writelines.\n", "Hope it's clear.\n"]
Next, open a file in either write ("w") or append mode to avoid overwriting existing content.
with open("example.txt", "w") as file:
file.writelines(lines)
In this scenario, "lines" represents a curated list of strings, each poised to be transcribed into the file "example.txt".
Take note of the newline symbol "\n" tagged at the end of each string, a crucial step to ensure each string is allocated its own line within the file.
The Advantages of Using writelines Over a Loop You might ponder the necessity of writelines over a traditional loop for writing each string individually with write. Although the difference in the outcome might seem negligible, writelines offers a streamlined, direct method, especially beneficial when you have a pre-prepared list of strings. It also edges out in efficiency by reducing the number of calls to the write function.
For a more intricate example, let's proceed.
Consider a scenario where you have a list of data, such as people's names, and your goal is to inscribe each on a new line within a file.
Initially, prepare your strings, then seamlessly integrate them with writelines.
# List of names
names = ["Alessia", "Giovanni", "Luca", "Martina"]
# Crafting the list of strings, appending '\n' to each name
lines = [f"{name}\n" for name in names]
# Executing the write operation
with open("names.txt", "w") as file:
file.writelines(lines)
In this example, a list comprehension technique is employed to affix the special character "\n" to each name, ensuring that every entry is distinguished on a new line when written to the file.
By leveraging writelines, we can write the entire list to 'names.txt' with a single, efficient command.