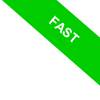
The readlines() Method in Python
When working with files in Python, there often comes a time when we need to read a file's entire contents to perform some operation on it. This is where the readlines() method steps in to make our lives easier.
readlines()
This handy function reads all the lines of a text file, returning them as a list of strings. Each string corresponds to a single line from the file.
Understanding How readlines() Works
Let's say you have a text file, "example.txt", filled with several sentences, each on a new line.
Here's how you could use readlines() to read this file:
with open('example.txt', 'r') as file:
lines = file.readlines()
Now, the variable "lines" contains a list where each element is a line from the file.
print(lines)
['First line\n', 'Second line\n', 'Third line']
Notice that each line, except the last one, ends with \n, which represents the "newline" character in many operating systems.
This is because readlines() includes the newline character at the end of each string in the list.
Why Is It Useful?
The readlines() method is particularly handy when you need to process a file line by line, whether it's for analysis or modification purposes.
For instance, to remove the newline character \n from each line, you could write:
with open('example.txt', 'r') as file:
lines = file.readlines()
clean_lines = [line.strip() for line in lines]
print(clean_lines)
This code strips away whitespace and newline characters from the start and end of each line, yielding a cleaner list.
['First line', 'Second line', 'Third line']
However, it's important to remember that readlines() reads the entire file into your computer's memory.
For very large files, this might not be the best approach.
If you're dealing with large files, consider reading the file line by line using a for loop, which is more memory-efficient.
For example, this code accomplishes the same task but reads one line at a time, allowing you to work on it without consuming too much memory.
with open('example.txt', 'r') as file:
for line in file:
print(line.strip())
['First line', 'Second line', 'Third line']
In summary, the readlines() method is a powerful tool for reading text files of moderate size, especially if you want to process the file's content as a list of lines.
Just be mindful of memory efficiency when working with very large files.