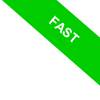
Python's seek() Method
Join us as we explore the seek() method in Python, a fundamental tool for file handling that enables precise control over the file’s read/write "cursor" position.
seek(offset, whence)
This method requires two arguments:
- offset is the number of bytes to move within the file.
- whence specifies the reference point for the offset. It can be one of three values:
- `0` or `SEEK_SET` starts from the beginning of the file. This is the default setting.
- `1` or `SEEK_CUR` moves from the current position.
- `2` or `SEEK_END` moves from the end of the file.
For text files, you can only use the second parameter set to 0 (from the beginning) or 2 (from the end) with an offset of 0. In other words, seek(0,2) is permitted, moving the cursor to the end of the file.
This method positions the file cursor to the location you specify.
After positioning the cursor, the seek() method also flushes the buffer.
How does it work? Picture having a book in front of you; the seek() method allows you to open the book to a specific page without flipping through each page individually.
Here's a practical example.
Imagine we have a text file named `esempio.txt`, containing the following:
Hello, this is a text file.
The second line starts here.
Third line, just another example.
Let's say you want to start reading from the sixth byte (character) in the file.
In this case, you would set the cursor to the sixth byte using seek(6) before reading the line.
with open('esempio.txt', 'r') as file:
# Move to the sixth character of the first line
file.seek(6)
content = file.readline()
print(content)
Python skips the first five characters, so it doesn't read "Hello," and prints the rest of the first line from the sixth character onwards.
this is a text file.
The seek method also allows you to jump back or forward a certain number of characters while reading the file.
For instance, to go back to the beginning of the file, you can use seek(0).
with open('esempio.txt', 'r') as file:
# Move to the sixth character of the first line
file.seek(6)
content = file.readline()
print(content)
# Move back to the start of the file
file.seek(0)
content = file.readline()
print(content)
In this scenario, Python reads the first line starting from the sixth character.
Then it moves the cursor back to position zero and reads the first line from the beginning of the file.
this is a text file.
Hello, this is a text file.
Using the read() method instead of readline(), you can read the entire content of the file starting from the sixth character.
with open('esempio.txt', 'r') as file:
# Move back to the start of the file
file.seek(6)
content = file.read()
print(content)
this is a text file.
The second line starts here.
Third line, just another example.
What about overwriting a file from a certain point?
Overwriting a file from a specific position is somewhat more complex since Python doesn't directly support writing in the middle of a file without overwriting existing content.
Typically, you would read the content into a data structure, modify it, and then rewrite the file.
When to Use seek(). The seek() method is especially useful when working with large files and you need to access specific parts without loading the entire file into memory. It’s also handy for binary files where you want to read or write data from/to specific locations.
We hope these practical examples have helped you understand how to utilize the seek() method in your Python projects!