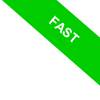
The open() Function in Python
Welcome to our guide on the open() function in Python, a cornerstone concept that every aspiring programmer needs to grasp. This tutorial will unpack the essentials, ensuring you have the knowledge to navigate file operations with ease.
open(name, mode)
At its core, the open() function is your gateway to interacting with files stored on your hard drive. It takes two primary arguments:
- File name (name): The name of the file you want to access. This is a required parameter.
- Mode of operation (mode): Here you specify the file's intended use—be it reading, writing, appending, or a combination. This parameter is optional and is represented by a string, such as `'r'` for reading, `'w'` for writing, `'a'` for appending, and `'r+'` for both reading and writing, among others.
The open() function generates a "file-object", serving as an iterator for seamless interaction with the file content.
This capability lets you effortlessly loop through the file object within a for loop, enabling you to read its contents line by line. It’s particularly useful when handling large files, as it sidesteps the need to load the full file into memory.
Overall, the open() function is versatile, offering the means to read, write new information, or add to existing files.
Note: If you do not specify the mode, Python defaults to read mode, allowing you to access a file's content without modifying it.
How to Open a File
Opening a file for reading is straightforward—simply use the mode 'r' for reading as the second argument in the open() function.
Let's say you have a text file named `esempio.txt` with several lines of text. Here's how you can open and read its contents:
file = open('example.txt', 'r')
content = file.read()
print(content)
file.close()
Once you've opened the file, the read() method lets you access its contents. Always remember to close the file with file.close() after you're done, much like you would close a book after reading it, to prevent any resource leaks.
Remember not to repurpose the label of a file object while it's in use. Python takes care of closing the file object for you as soon as it's no longer associated with its initial identifier or reference label. This process includes flushing the buffer and releasing the memory space that was allocated. Take, for example, this script that opens a file and assigns it the label "myFile".
myFile = open('example.txt', 'w')
myFile = 10
Latter, when the script assigns a new value of 10 to "myFile", Python automatically steps in to close the file object that was linked to "myFile". This happens because the original identifier/label is no longer pointing to the file object.
Writing to a File
To write to a file, switch to the mode 'w' for writing. A word of caution: this action will overwrite any existing content if the file already exists.
file = open('new.txt', 'w')
file.write('Hello, world!')
file.close()
The write() method allows you to pour new content into the file. If 'new.txt' doesn't exist, Python will create it. If it does, it will be refreshed with the new content you provide.
Remember that when using the open() function for writing, it operates with a buffering mechanism. This essentially means that the write() method doesn't directly commit changes to the file but temporarily stores them in memory. Therefore, it's essential to ensure that the buffer is emptied at the end of your operation to guarantee that all your data is correctly written to the file. You can achieve this by either invoking the flush() method or by securely closing the file using the close() method.
Appending Content to a File
To add to a file without erasing its existing content, opt for the mode 'a' for appending.
file = open('example.txt', 'a')
file.write('\nAnother line of text.')
file.close()
This mode ensures that if the file already exists, your new content is seamlessly added to the end, preserving what was already there. If the file doesn’t exist, Python takes the initiative to create it and then appends the content.
The Elegance of Using with
Python offers a more sophisticated approach to file handling with the with statement, automating the file closure process. It’s akin to entrusting Python with both opening and closing the file, simplifying your code and ensuring no file is left open accidentally.
with open('example.txt', 'r') as file:
content = file.read()
print(content)
With this method, there's no need to explicitly close the file. Python handles it for you once you exit the `with` block, making your code cleaner and more error -proof. Just remember to properly indent your code within the with block to maintain clarity and structure.