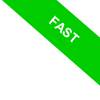
Python's tell() Method
Let's dive into the tell() method in Python—a key function for file objects that provides the current position of the cursor within a file.
file.tell()
Imagine the cursor as a marker that advances each time you perform read or write operations on the file.
This knowledge is crucial for efficiently navigating through large files or when precise manipulation of file content is required.
How Does tell() Work? Opening a file with Python's open() function sets you at the beginning, or position 0, of the file. As you read or write, the cursor moves a number of bytes equal to the characters processed. The tell() method reveals the cursor's current location.
Let's clarify with an example:
Suppose you have a text file named example.txt with the following content:
Hello, world!
Welcome to the example file.
This is how tell() can be utilized to track our position while reading through the file:
# Open the file in read mode
with open('example.txt', 'r') as file:
# Read the first five characters
first_chunk = file.read(5)
# Use tell() to check our position
print("Current cursor position:", file.tell())
# Read the next five characters
second_chunk = file.read(5)
# Verify the cursor's new location
print("Cursor position now:", file.tell())
Initially, the cursor position is at 5, after reading "Hello,". Following the next set of five characters, " world", the cursor moves to position 10.
The Practicality of tell()
Why is the tell() method so valuable?
- It facilitates returning to a specific file position after various read/write operations, utilizing tell() to mark your spot and seek() to return.
- It is indispensable for working with binary files, where manipulating specific bytes is often necessary.
- It aids in debugging, ensuring data is accurately read from or written to the intended section of the file.
A critical reminder: managing file resources with care is paramount. Always close files with the close() method after use, or employ the with statement, which automatically handles closure post-operation, as demonstrated above.
In wrapping up, the tell() method is an essential tool for precise file manipulation. Through practical examples, I aim to have made its utility both clear and easily graspable.