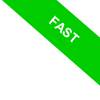
Python Tuple Methods
In Python, tuples serve as immutable data structures. This immutability implies that once a tuple is defined, its state remains unchanged; it can't be modified. Because of this characteristic, tuples do not boast a multitude of methods as their counterparts - lists and dictionaries do. Nevertheless, there are a couple of fundamental methods associated with tuples:
The count() Method
This method is employed to determine the frequency of a particular value within the tuple.
Consider the following example where we create a tuple:
tuple = (1, 2, 2, 3, 3, 3)
We then proceed to use the count() method to ascertain how many times the number "2" appears in our tuple.
print(tuple.count(2))
In this case, we find that the number "2" appears twice in the tuple.
2
The index() Method
This method returns the position of the first occurrence of a particular value in the tuple. If the value isn't present, the method raises an error.
Let's illustrate this with an example where we again create a tuple.
tuple = (1, 2, 2, 3, 3, 3)
We then search for the first instance of the number "2" in our tuple.
print(tuple.index(2))
In this scenario, we see that the first instance of the number "2" is located at index position 1.
1
These are the essential methods specific to Python tuples.
For all other operations involving tuples, you can rely on Python's general-purpose functions and operators that work with sequence types. These include, but are not limited to, len(), +, and *.