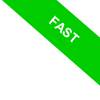
Python Tuple index() Method
Python's index() method serves as a handy tool for locating an element's initial occurrence within a tuple. Essentially, it is used as follows:
tuple.index(element, start, end)
This method takes in three parameters:
- The element you're aiming to find within the tuple
- The 'start' point in the index where you intend to kick off your search
- The 'end' point in the index where you wish to wrap up your search
Once invoked, the index method will diligently return the position of the sought element within the tuple's index.
If, however, it fails to spot the element in the tuple, an exception is raised, and an error message is displayed for your attention.
Worth noting is that the 'start' and 'end' positions are optional parameters for the search. If you decide not to specify them, the index() method will conveniently embark on a comprehensive search across the entirety of the tuple.
To help illustrate this, let's consider a practical example.
Suppose we create a tuple in the variable "numbers":
numbers = (1, 2, 3, 4, 3, 2, 1, 2, 3)
Now, we employ the numbers.index(3) method to spot the first occurrence of "3" in the tuple:
print(numbers.index(3))
The tuple indeed houses three elements valued "3".
As per its mandate, the method will return the position of the first occurrence of this value, which, in our case, is at index position 2.
2
It's crucial to bear in mind that Python's indices start from zero. Thus, the first element will be at index 0, the second at index 1, and so forth.
Next, let's try to find the first occurrence of the element "3", but this time, our search will commence from the index position 5.
print(numbers.index(3,5))
In this scenario, the numbers.index(3,5) method starts the hunt from the sixth element of the tuple (index position 5).
The index() method subsequently uncovers the first "3" at position 8.
8
Lastly, let's run the following command:
print(numbers.index(3, 5, 7))
The numbers.index(3,5,7) method attempts to locate the first "3" in the tuple between index positions 5 and 7.
Interestingly, in this case, the index() method returns a ValueError exception. It appears that there's no element equal to "3" within the selected range.
ValueError: 3 is not in tuple
Thus, the index() method in Python provides a robust way to find elements within a tuple, from any specified range, while handling exceptions effectively.