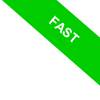
Python's deepcopy Method
The deepcopy() method in Python is a powerful tool for duplicating iterable objects like dictionaries and lists, ensuring that no external links or references are copied. Here's a quick overview of how it works:
copy.deepcopy(x, memo=None, _nil=[])
- x is the object you're duplicating. This can be any Python object, including, but not limited to, dictionaries, lists, and custom classes.
- memo (optional) is a dictionary that the deepcopy() function uses to avoid infinite recursion in cases of circular references. While it's a crucial part of deepcopy's internal mechanics, it's rarely necessary for users to specify this parameter.
- _nil (optional) is a technical parameter for internal use within the deepcopy() function. It's typically not modified or directly used in everyday scripting.
This method ensures that a copy of an object includes its data, not just references to the original.
Why Use deepcopy? DeepCopy is indispensable when you're dealing with objects that include mutable elements like dictionaries and lists. By using deepcopy, you ensure a thorough duplication of the data, thereby preventing the copied links from affecting the original objects. Thus, any changes you make to a copied list or dictionary won't impact the original version.
Let’s dive a bit deeper into the difference between shallow and deep copying:
- Shallow Copy: This approach creates a new instance of the original object but keeps the references to the contained objects. As a result, any modifications to the contents of the copy will also appear in the original.
- Deep Copy: In contrast, a deep copy generates a completely independent clone of the original object, including all nested objects. Changes made to the deep copy won't affect the original in any way.
Keep in mind, before using deepcopy, you need to import it from Python's copy module:
import copy
Now that we've covered the basics, let's explore some practical applications of the deepcopy() method with dictionaries and lists.
Exploring the deepcopy() Method with Python Dictionaries
Begin by creating a dictionary, each key paired with its respective values.
original_dictionary = {'key1': [1, 2, 3], 'key2': {'subkey': 4}}
Then, execute a deep copy of this dictionary using the deepcopy() method.
copied_dictionary = copy.deepcopy(original_dictionary)
Next, let's modify the deep copied dictionary. For instance, add the number 4 to the list under key1.
copied_dictionary['key1'].append(4)
Proceed to reveal the contents of the deep copied dictionary.
print("Deep Copy:", copied_dictionary)
Notice the dictionary now reflects the recent addition.
Deep Copy: {'key1': [1, 2, 3, 4], 'key2': {'subkey': 4}}
It's also essential to check the original dictionary.
print("Original:", original_dictionary)
The original dictionary remains intact and unaffected.
Original: {'key1': [1, 2, 3], 'key2': {'subkey': 4}}
Had a shallow copy been made, the original dictionary would have been altered as well.
Shallow Copy Illustration: To highlight the contrast, let's replicate the scenario with a shallow copy. Redefine the original dictionary.
original_dictionary = {'key1': [1, 2, 3], 'key2': {'subkey': 4}}
Create a shallow copy using the copy method.
shallow_copied_dictionary = original_dictionary.copy()
Modify an element in the shallow copy, such as adding the number 4 to the list associated with key1.
shallow_copied_dictionary['key1'].append(4)
Now, display the contents of both the shallow copy and the original dictionary.
print("Shallow Copy:", shallow_copied_dictionary)
print("Original:", original_dictionary)
In this scenario, the original dictionary is also impacted by the modification, unlike with the deep copy.
Shallow Copy: {'key1': [1, 2, 3, 4], 'key2': {'subkey': 4}}
Original: {'key1': [1, 2, 3, 4], 'key2': {'subkey': 4}}
This example effectively demonstrates the stark difference between a deep copy, executed via deepcopy, and a shallow copy achieved with the copy method.
Understanding the Deepcopy() Method with Lists
Consider a scenario where you're working with a nested list - a list that includes other lists within it.
original_list = [1, [2, 3], [4, 5]]
This particular list is a mix of simple elements, like numbers, and more complex ones, such as sub-lists.
Now, suppose you need to duplicate this list, ensuring that any modifications to the clone won't impact the original. This is where deepcopy() comes into play.
By employing deepcopy(), you can create a thoroughly independent copy of the original list.
copied_list = copy.deepcopy(original_list)
Let's tweak an element in the copied list as an example. Say, you add the number 6 to the sub-list [2, 3], located in the second slot of the copied list.
copied_list[1].append(6)
Then, take a look at the contents of both lists.
print("Original List:", original_list)
print("Copied List:", copied_list)
Notice how the changes are confined to the copied list, leaving the original untouched.
Original List: [1, [2, 3], [4, 5]]
Copied List: [1, [2, 3, 6], [4, 5]]
In contrast, a shallow copy would have resulted in the original list being altered as well.
Example of a Shallow Copy: To illustrate the difference, let’s perform a similar operation using a shallow copy. Start by defining a list.
original_list = [1, [2, 3], [4, 5]]
Execute a shallow copy using the copy method.
copied_list = original_list.copy()
Modify an element in the sub-list of the copied version. For instance, append the value 6.
copied_list[1].append(6)
Finally, compare the two lists after this modification.
print("Original List:", original_list)
print("Copied List:", copied_list)
Here, the alteration in the copied list also affects the original, highlighting the difference between shallow and deep copying.
Original List: [1, [2, 3, 6], [4, 5]]
Copied List: [1, [2, 3, 6], [4, 5]]
Deepcopy Method in Tuples
Let's delve into the application of the deepcopy method within tuples.
Consider a tuple that includes both immutable and mutable elements. For example:
original_tuple = (1, 2, 3, [4, 5])
This tuple is a mix of straightforward elements like numbers 1, 2, and 3, and a more complex element such as the list [4,5].
Next, apply deepcopy() to create an exact, deep copy of this tuple.
copied_tuple = copy.deepcopy(original_tuple)
Alter the mutable element within the copied tuple – the list [4,5] – by appending the number 6.
copied_tuple[3].append(6)
Now, let's compare the original and the modified copies:
print("Original:", original_tuple)
print("Copy:", copied_tuple)
Notice how altering the mutable element in the copied tuple leaves the original tuple unaffected.
Original: (1, 2, 3, [4, 5])
Copy: (1, 2, 3, [4, 5, 6])
These examples succinctly showcase the effectiveness of deepcopy() in maintaining the separation and integrity of original data structures, even when substantial alterations are made to their duplicates.