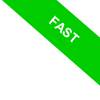
Named Tuples in Python
In the Python programming language, named tuples offer a clear and structured way to handle data, with each element possessing both a name and a value. This feature not only enhances code readability but also simplifies data management.
Exploring Named Tuples View named tuples as an advanced iteration of standard tuples. A tuple, as you may know, is an immutable sequence of values. Once created, its content cannot be altered. Accessing tuple elements is typically done using numeric indices, like tupla[0] or tupla[1].
tupla[0]
However, relying solely on numeric indices can be confusing and hard to track. Named tuples elegantly solve this issue. They function similarly to regular tuples but with a significant enhancement: each element is named. For example, elements can be accessed with tupla.name or tupla.year, lending clarity and ease of use to your code.
tupla.name
Creating them is straightforward, using the namedtuple class from the collections module.
Why Use Named Tuples?
Named tuples are instrumental in crafting clean, understandable code. They shine particularly in scenarios involving extensive data, where code organization and readability are paramount, not just for you but also for others who might read your code.
Crafting a Named Tuple
Start by importing the namedtuple class from the collections module.
from collections import namedtuple
Then, define your namedtuple, which is akin to a simplified class definition.
Person = namedtuple('Person', ['name', 'age'])
Here, we 've created a namedtuple called "Person" with two attributes: "name" and "age".
Alternatively, you can define the fields of your namedtuple in a single string, using either spaces or commas for separation, instead of a list.
Person = namedtuple('Person', 'name age')
This approach achieves the same outcome.
Now, let's instantiate the namedtuple "Person".
person1 = Person(name="Alessandro", age=30)
This creates an instance of "Person" with the name Alessandro and age 30. This method of element access, using names instead of indices, is intuitively easier and more efficient.
Accessing Tuple Fields
To view the list of fields in your namedtuple, simply use the __fields property.
print(person1._fields)
('name', 'age')
Element Access in Named Tuples
With named tuples, element access is based on names rather than numeric indices. For instance, to view the name of the instance person1, you would use:
print(person1.name)
Alessandro
Similarly, accessing the age is as straightforward as referring to the age field.
print(person1.age)
30
This method is far more intuitive and user-friendly than traditional index-based access.
Immutability. As with standard tuples, named tuples are immutable. Once set, their values cannot be altered, which helps avoid unintentional data modifications. However, you can generate a modified copy of an existing tuple using the __replace() method, a topic we'll delve into later.
Practical Application
Consider, for example, representing a point in a 2D space:
Point = namedtuple('Point', ['x', 'y'])
p = Point(11, y=22)
print(p.x, p.y)
11
22
Or, if managing vehicle data is your task:
Car = namedtuple('Car', 'make model year color')
my_car = Car('Fiat', '500', 2015, 'blue')
print(my_car.make)
Fiat
Updating with _replace()
Despite their immutable nature, named tuples offer flexibility through the _replace() method. This allows you to create a new tuple with modified values.
person2 = person1._replace(age=31)
print(person2.age)
31
It's important to note that the original tuple, person1, remains unaltered.
print(person1.age)
30
The _asdict() Method
Discover how the _asdict() method effortlessly transforms a namedtuple into an OrderedDict.
from collections import namedtuple
Person = namedtuple('People', ['name', 'age'])
person1 = Person(name="Alessandro", age=30)
print(person1._asdict())
{'name': 'Alessandro', 'age': 30}
Merging namedtuples and Tuples in Python
Explore how you can merge a namedtuple with a tuple in Python, creating a seamless combination.
The end result will be a unified tuple.
from collections import namedtuple
Person = namedtuple('People', ['name', 'age'])
person1 = Person(name="Alessandro", age=30)
tuple=(1,2)
print(person1+tuple)
('Alessandro', 30, 1, 2)
This guide aims to provide you with a deeper understanding of namedtuples in Python, enhancing your coding toolkit.