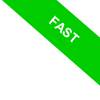
Creating a Numeric Sequence in an Array with Matlab
In this lesson, I will teach you how to create a sequence of ascending or descending numbers in an array variable using Matlab.
To create a numerical sequence, you need to use three parameters: the beginning of the sequence (START), the step, which is the increment or decrement of the sequence (STEP), and the end of the sequence (STOP). Separate the parameters with a colon symbol. The numerical sequence is defined with START, STEP, and STOP.
Information about the step is optional. When it's missing, Matlab uses a default step of +1. Alternatively, you can also use the functions linspace() and logspace().
Let me give you a practical example.
To create an array with a sequence of integer numbers from 1 to 10, type v=1:10.
>> v=1:10
v =
1 2 3 4 5 6 7 8 9 10
In the previous command, the step is not defined. The sequence is only defined by the START=1 and STOP=10 parameters.
Matlab processes the command with a unit step as if it were v=1:1:10.
>> v=1:1:10
v =
1 2 3 4 5 6 7 8 9 10
Now create an array composed only of odd integers.
Type the command v=1:2:10 with START=1 and STEP=2.
>> v=1:2:10
v =
1 3 5 7 9
Next, create an array composed only of even integers.
Type the command v=2:2:10, where the step is always STEP=2, but the beginning is START=2.
>> v=2:2:10
v =
2 4 6 8 10
To have a decreasing sequence, indicate a higher starting value START than the ending value STOP and a negative step STEP=-1.
For example, type the command v=10:-1:1.
>> v=10:-1:1
v =
10 9 8 7 6 5 4 3 2 1
This command also allows you to define a sequence of real values.
For example, type the command v=0:0.2:1 to create a sequence of real numbers from 0 to 1 with a decimal step STEP=0.2.
>> v=0:0.2:1
v =
0.00000 0.20000 0.40000 0.60000 0.80000 1.00000
Then create a sequence of real numbers from -1 to 1 by setting the step to STEP=0.5.
Type the command v=-1:0.5:1
>> v=-1:0.5:1
v =
-1.0000 -0.5000 0.0000 0.5000 1.0000
This syntax allows you to create any numerical sequence of integers or real numbers.
Note. This syntax has limitations, however. For example, it doesn't allow you to create sequences of complex numbers. If you want to get a sequence of complex numbers, you need to use the linspace() function.
The linspace() function creates a numerical sequence of integers, real, or complex numbers. It's a more complete function than the previous one.
linspace(start, stop, n)
- The first parameter (start) is the initial value of the numerical sequence.
- The second parameter (stop) is the final value of the numerical sequence.
- The parameter n is the number of elements in the sequence.
Let me give you a practical example.
Type v=linspace(1,10,5) to create a sequence of real numbers ranging from 1 to 10, comprising only 5 elements.
For instance, the output of this code would be:
>> v=linspace(1,10,5)
v =
1.0000 3.2500 5.5000 7.7500 10.000
Furthermore, the linspace() function also enables you to generate a sequence of complex numbers.
To illustrate, you can create a sequence of three complex numbers between 1+2i (starting point) and 3+4i (ending point).
Here is an example code:
>> v=linspace(1+2i, 3+4i, 3)
v =
1 + 2i 2 + 3i 3 + 4i
If you wish to create a sequence of numbers in a logarithmic scale, you can use the logspace() function.
logspace(a,b,n)
- The first parameter represents the exponent of the starting point, which is 10a
- The second parameter denotes the exponent of the ending point, which is 10b
- The third parameter, n, signifies the number of elements within the sequence.
As an example, typing logspace(1,2,5) will produce a sequence of 5 values, ranging within the logarithmic scale between 101 and 102 (that is, between 10 and 100).
>> logspace(1,2,5)
ans =
10.000 17.783 31.623 56.234 100.000