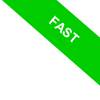
Functions of Arrays in Matlab
Matlab provides several functions that simplify calculations on arrays, saving you a lot of time once you familiarize yourself with them.
Let me give you some practical examples.
Create an array and assign it to a variable called 'vector'.
>> vector = [1 4 2 6 3]
vector =
1 4 2 6 3
Here are some particularly useful methods you can use:
sum()
Calculates the sum of the elements in the array.
>> sum(vector)
ans = 16
It's a simple algebraic sum of the values in the array: $$ 1 + 4 + 2 + 6 + 3 = 16 $$
prod()
Multiplies the elements in the array.
>> prod(vector)
ans = 144
Calculates the product of the elements in the array. $$ 1 \cdot 4 \cdot 2 \cdot 6 \cdot 3 = 144 $$
length()
Returns the number of elements in the array, i.e., the length of the vector or the number of elements in a matrix.
>> length(vector)
ans = 5
In this case, the array variable consists of five elements: [ 1 4 2 6 3 ]
mean()
Calculates the arithmetic mean of the elements in the array.
>> mean(vector)
ans = 3.2000
The arithmetic mean of the elements in the vector is ( $$ \frac{1+4+2+6+3}{5} = \frac{16}{5} = 3.2 $$
max()
Finds the maximum value among the elements in the array.
>> max(vector)
ans = 6
The maximum value in the array [1 4 2 6 3] is 6.
min()
Finds the minimum value among the elements in the array.
>> min(vector)
ans = 1
The minimum value in the array [ 1 4 2 6 3 ] is 1.
find()
Finds the positions of the elements in the array that satisfy a selection criterion.
>> find(vector > 2)
ans =
2 4 5
In this case, the elements greater than 2 in the array[ 1 4 2 6 3 ] are located at positions 2, 4, and 5, which correspond to the second, fourth, and fifth elements of the array.
sort()
Sorts the elements of the vector in ascending or descending order.
>> sort(vector, 'ascend')
ans =
1 2 3 4 6
or in descending order
>> sort(vector, 'descend')
ans =
6 4 3 2 1
The second parameter is 'ascend' by default.
So, if you only type the function sort(vector) without specifying the second parameter, Matlab will default to sorting the elements in ascending order.
>> sort(vector)
ans =
1 2 3 4 6
round()
Rounds the elements of the array to the nearest integer value.
>> vector = [0.2 -0.4 1.4 1.9 -2.1]
vector =
0.20000 -0.40000 1.40000 1.90000 -2.10000
>> round(vector)
ans =
0 -0 1 2 -2
fix()
Rounds the elements of the array to the nearest integer value towards zero.
>> vector = [ 0.2 -0.4 1.4 1.9 -2.1 ]
vector =
0.20000 -0.40000 1.40000 1.90000 -2.10000
>> fix(vector)
ans =
0 -0 1 1 -2
floor()
Rounds the elements of the array to the nearest integer towards negative infinity (to the left of the number).
>> vector = [ 0.2 -0.4 1.4 1.9 -2.1 ]
vector =
0.20000 -0.40000 1.40000 1.90000 -2.10000
>> floor(vector)
ans =
0 -1 1 1 -3
ceil()
Rounds the elements of the array to the nearest integer towards positive infinity (to the right of the number).
>> vector = [ 0.2 -0.4 1.4 1.9 -2.1 ]
vector =
0.20000 -0.40000 1.40000 1.90000 -2.10000
>> ceil(vector)
ans =1 -0 2 2 -2
These functions are particularly useful as they simplify calculations on arrays.