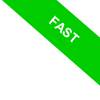
Searching for a Value in an Array
In this guide, I will explain how to search for a value in a MATLAB array using the find() function.
find(X,N,D)
The function has three parameters:
- X
The parameter X is an array and is a required parameter. - N
The parameter N is an integer that indicates the maximum number of elements to be found. The search is terminated when this number is reached. - D
The parameter D is the direction of the search. It can be "first" to start the search from the first element of the index, or "last" to start it from the last element.
The function returns the indices of the elements in the array that satisfy the search.
Note. The indices of arrays are always sorted in ascending order, even when the search starts from the last element.
Let me give you a practical example.
Create a one-dimensional array.
>> V = [ 1 0 2 0 4 0 4 ]
V =
1 0 2 0 4 0 4
This array has seven elements.
In MATLAB, the index of elements in an array starts from one.
Enter find(V) to find the non-zero elements.
>> find(V)
ans =
1 3 5 7
The function returns the positions where the non-zero elements are located, i.e., V[1], V[3], V[5], and V[7].
Note. The same applies even if the array is arranged in rows instead of columns. The find() function returns the positions in the index.
To find only the first element that satisfies the condition, enter 1 as the second parameter.
Enter find(V,1)
>> find(V,1)
ans = 1
The function returns the index of the first non-zero element in the array, i.e., V[1].
To search for elements starting from the last, indicate "last" as the third parameter.
Enter find(V,1,"last")
>> find(V,1,"last")
ans = 7
In this case, the search direction is from right to left.
The first element that satisfies the condition is V[7].
To search for elements equal to zero, put a negation in front of the array name.
Enter find(~V)
>> find(~V)
ans =
2 4 6
The function returns the positions where the elements in the array are equal to zero, i.e., V[2], V[4], and V[6].
To identify only the elements in the array that are greater than two, enter find(V>2)
>> find(V>2)
ans =
5 7
The function returns the elements V[5] and V[7] of the array.
If the search does not select any element in the array, the result is an empty set.
For example, enter find(V>5) to search for elements greater than 5.
The result is an empty array because the largest value in the array is 4.
>> find(V>5)
ans =
1×0 empty double row vector
To search for elements in the array with values between 1 and 3, enter find(V<=3 & V>=1)
>> find(V<=3 & V>=1)
ans =
1 3
The elements that satisfy the condition are V[1] and V[3].
What about multidimensional arrays?
When dealing with multidimensional arrays, such as matrices, the find function searches through the columns one by one, starting from the first column.
For example, let's create a 3x3 matrix
>> M=[1 0 1; 0 1 0; 1 0 1]
M =
1 0 1
0 1 0
1 0 1
This matrix has two dimensions with three rows and three columns
$$ \begin{pmatrix} 1 & 0 & 1 \\ 0 & 1 & 0 \\ 1 & 0 & 1 \end{pmatrix} $$
To find the indices of the non-zero elements, you can type find(M)
>> find(M)
ans =
1
3
5
7
9
Indices 1 and 3 correspond to the first two non-zero elements in the first column.
Index 5 corresponds to the non-zero element in the second column, which is the fifth element in the sequence.
Indices 7 and 9 correspond to the non-zero elements in the third column, which are the seventh and ninth elements in the sequence.
In essence, the find() function returns the position of the elements in the matrix as if they were part of a single vector.
To obtain the position of the elements in the matrix with row and column coordinates, you can type [row,col,v]=find(M)
>> [row,col]=find(M)
row =
1
3
2
1
3
col =
1
1
2
3
3
v =
1
1
1
1
1
The function extracts three arrays, row and col, which contain the row and column coordinates of the elements, respectively. Another array, v, contains the values.
To view the coordinates of the first selected element, you can type row(1),col(1)
>> row(1),col(1)
ans = 1
ans = 1
The first element is located at coordinates (1,1) on the first row and first column.
To view the coordinates of the second selected element, you can type row(2), col(2)
>> row(2),col(2)
ans = 3
ans = 1
The second element is located at coordinates (3,1) on the third row and first column.
This way, you can determine the position of the elements in the matrix accurately.