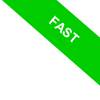
How to Define Vectors in Matlab
In this lesson, I will explain how to create a vector in Matlab.
Let's start with a practical example.
Consider a vector on the plane that originates from the point (0;0) and points to the coordinates v(2;4).
The vector has two components, x=2 and y=4.
To define this vector in Matlab, you need to use a two-dimensional array variable.
In the array, separate the x and y components of the vector using a semicolon.
>> v=[2;4]
This way you define a column vector.
$$ \vec{v} = \begin{pmatrix} x \\ y \end{pmatrix} = \begin{pmatrix} 2 \\ 4 \end{pmatrix} $$
You can use the array you just created in any vector computation operation (e.g., vector addition, cross product, dot product, etc.).
How to Create a Three-Dimensional Vector in Matlab
To create a vector with three or more components, you can follow the same procedure.
For example, consider a three-dimensional vector (in 3D space) that points to the coordinates v(2;4;3).
This vector has three components, x=2, y=4, z=3.
$$ \vec{v} = \begin{pmatrix} x \\ y \\ z \end{pmatrix} = \begin{pmatrix} 2 \\ 4 \\ 3 \end{pmatrix} $$
To define this vector in the Matlab environment, create an array with three components.
>> v=[2;4;3]
How to Create a Row Vector
So far, I have explained how to create a column vector where the elements are arranged vertically.
$$ \vec{v} = \begin{pmatrix} a \\ b \\ c \end{pmatrix} $$
If you need to create a row vector, where the elements are arranged horizontally, you need to use a different syntax.
$$ \vec{v} = \begin{pmatrix} a & b & c \end{pmatrix} $$
To create a row vector in Matlab, create an array and separate the components with a space or a comma.
For example, to define this row vector:
$$ \vec{v} = \begin{pmatrix} 1 & 2 & 3 \end{pmatrix} $$
Type an array and separate the elements with a comma.
>> v=[1, 2, 3]
The result is a row vector.
Note. Alternatively, you can create a column vector v=[1;2;3] and convert it into a row vector by transposing v