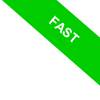
How to extract elements from an array in Matlab
In this lesson, I'll explain how to extract elements from a Matlab array using the slicing technique.
First, create an array with some numerical values.
>> v=[10 11 12 13 14 15 16]
v =
10 11 12 13 14 15 16
The array is composed of seven elements, which in this case are seven integers.
If you want to extract the first three elements of the array, type b=v(1:3)
>> b=v(1:3)
b =
10 11 12
This command extracts the elements from position 1 (the first element) to position 3 (the third element) of array v.
In Matlab, the first element of an array always has an index equal to one.
Then save the newly extracted values in array b.
Note that the colon symbol (:) separates the two extreme positions of the range. This technique is called slicing and allows you to extract and modify the array quickly and easily. It's also used in other programming languages, such as Python.
If you want to extract the third, fourth, and fifth elements of array v, type b=v(3:5)
>> b=v(3:5)
b =
12 13 14
To extract the first, third, and fifth values of array v, type b=v([1 3 5])
>> b=v([1 3 5])
b =
10 12 14
You can also extract two intervals of elements from the array by separating them with a space or a comma.
For example, to extract the first and second elements along with the sixth and seventh elements, type b=v([1:2 6:7])
>> b=v([1:2 6:7])
b =
10 11 15 16
To extract the last element of the array, you can indicate the position of the element or use the keyword "end."
For example, to extract the last element of array v, type b=v(end)
>> b=v(end)
b = 16
The keyword end is very useful because it allows you to extract the last or last few elements of the array even if you don't know the length of the array or the number of its elements.
For example, if you want to extract the elements of the array from the fourth element onwards, type b=v(4:end)
>> b=v(4:end)
b =
13 14 15 16
If you want to extract the last three elements of the array, type b=v(end-2:end)
>> b=v(end-2:end)
b =
14 15 16
You can also extract elements by setting a step size and adding an additional intermediate parameter in the slicing.
What is the step size? The step size is an integer that indicates the increment from one position of the array to the next position during extraction.
For example, to extract the elements in odd positions, type b=v(1:2:end)
In this case, Matlab extracts the elements from the first to the last using a step size of 2.
>> b=v(1:2:end)
b =
10 12 14 16
The step size can also be a negative integer.
For example, to extract the elements of the array from the last to the first in reverse order, type b=v(end:-1:1)
>> b=v(end:-1:1)
b =
16 15 14 13 12 11 10
Slicing also allows you to replace some elements of the array by assigning them other values.
For example, to replace the first and second values of the array with 20 and 21, type v([1 2]) = [20 21]
>> v([1 2]) = [20 21]
v =
20 21 12 13 14 15 16
You can also assign a single value to multiple elements of the array.
For example, to assign the value 99 to the first and second element of the array, type v([1 2]) = 99
>> v([1 2]) = 99
v =
99 99 12 13 14 15 16
This allows you to easily modify specific elements of an array using a single command.