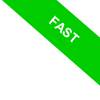
Sorting an Array in Matlab
In this lesson, I will explain how to sort elements in an array (vector) using Matlab.
Let's consider a practical example.
Create an array with five elements.
>> v = [ 4 2 1 6 3 5 ]
v =
4 2 1 6 3 5
The elements in the array are integers arranged in an unordered sequence.
To sort the elements in the array in ascending order, use the sort(v) function.
>> sort(v)
ans =
1 2 3 4 5 6
The sort(v) function arranges the elements in the array from smallest to largest.
To sort the elements in the array in descending order, use the sort() function and add the 'descend' parameter.
>> sort(v, 'descend')
ans =
6 5 4 3 2 1
Now the elements in the array are sorted from largest to smallest.
The sort() function also allows you to sort multidimensional arrays.
For example, create a 3x3 matrix.
>> M = [ 1 4 1 ; 2 8 3 ; 5 1 6 ]
M =
1 4 1
2 8 3
5 1 6
A matrix is a two-dimensional array because the elements are arranged in rows and columns.
To sort the elements in the matrix, simply type sort(M).
>> sort(M)
ans =
1 1 1
2 4 3
5 8 6
The sort(M) function returns a matrix with all elements sorted from smallest to largest.
If you use the keyword "descend" to sort a matrix, Matlab treats each column of the matrix as a vector and sorts its elements.
For example, type sort(M,"descend").
>> sort(M)
ans =
5 8 6
2 4 3
1 1 1
In this case, Matlab sorts the columns of the matrix separately.
For instance, consider a scenario where the first column of an array contains the elements 1, 2, and 5. In Matlab, these elements would be sorted in vertical order as 5, 2, and 1. Similarly, if the second column of the matrix comprises the elements 4, 8, and 1, Matlab would sort them in descending order, resulting in a vertical arrangement of 8, 4, and 1. This pattern continues for subsequent columns and elements.