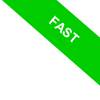
Vector Operations in Matlab
In this lesson, I will explain the main vector calculations in MATLAB using simple practical examples.
First, define a vector.
>> v=[1; 3; 4;]
Then define another vector in the same space.
>> w=[2; 1; -1]
The two vectors have three components arranged vertically, so they are column vectors in three-dimensional space (x, y, z).
Note. In these examples, I have asked you to define column vectors, but you can also define row vectors. Vector calculations are the same regardless of whether the vectors are row or column vectors.
Here are some mathematical operations you can perform with the two vectors in MATLAB.
Addition of Vectors
The operator for addition between two vectors is the plus sign (+).
To add the two vectors in MATLAB, type v + w
>> v+w
ans =
3
4
3
$$ \vec{v} + \vec{w} = \begin{pmatrix} 1 \\ 3 \\ 4 \end{pmatrix} + \begin{pmatrix} 2 \\ 1 \\ -1 \end{pmatrix} = \begin{pmatrix} 1+2 \\ 3+1 \\ 4-1 \end{pmatrix} = \begin{pmatrix} 3 \\ 4 \\ 3 \end{pmatrix} $$
Subtraction Vectors
The operator for subtraction between two vectors is the minus sign (-).
To obtain the difference between two vectors, type v - w
>> v-w
ans =
-1
2
5
$$ \vec{v} - \vec{w} = \begin{pmatrix} 1 \\ 3 \\ 4 \end{pmatrix} - \begin{pmatrix} 2 \\ 1 \\ -1 \end{pmatrix} = \begin{pmatrix} 1-2 \\ 3-1 \\ 4-(-1) \end{pmatrix} = \begin{pmatrix} -1 \\ 2 \\ 5 \end{pmatrix} $$
Vector Dot Product
The operator for multiplication between two vectors is the asterisk sign (*).
In this case, you have defined two column vectors. Therefore, to calculate the dot product of the two vectors, you need to transpose one of the vectors into a row vector.
To transpose a vector, add an apostrophe to the right of the array name.
For example, multiply the first vector v by the transpose of the second vector w'
>> v*w'
ans =
2 1 -1
6 3 -3
8 4 -4
$$ \vec{v} \cdot \vec{w}^T = \begin{pmatrix} 1 \\ 3 \\ 4 \end{pmatrix} \cdot \begin{pmatrix} 2 & 1 & -1 \end{pmatrix} = \begin{pmatrix}1 \cdot 2 & 1 \cdot 1 & 1 \cdot (-1) \\3 \cdot 2 & 3 \cdot 1 & 3 \cdot (-1) \\ 4 \cdot 2 & 4 \cdot 1 & 4 \cdot (-1) \end{pmatrix} = \begin{pmatrix}2 & 1 & -1 \\ 6 & 3 & -3 \\ 8 & 4 & -4 \end{pmatrix} $$
You can also transpose the first vector v' and multiply it by the second vector w.
However, remember that vector multiplication does not obey the commutative property. So, the product v'*w is different from the product v*w'.
>> v'*w
ans = 1
$$ \vec{v}^T \cdot \vec{w} = \begin{pmatrix} 1 & 3 & 4 \end{pmatrix} \cdot \begin{pmatrix} 2 \\ 1 \\ -1 \end{pmatrix} = \begin{pmatrix} 1 \cdot 2 + 3 \cdot 1 + 4 \cdot (-1) \end{pmatrix} = \begin{pmatrix} 2 + 3 - 4 \end{pmatrix} \begin{pmatrix} 1 \end{pmatrix} = 1 $$
Element-wise multiplication
Element-wise multiplication is another type of vector multiplication.
In this case, the operation calculates the product of the elements of the two vectors that are in the same position.
To perform element-wise multiplication in Matlab, use the symbol .* (dot and asterisk).
>> v.*w
ans =
2
3
-4
In element-wise multiplication, both vectors v and w must be either row vectors or column vectors.
Furthermore, vectors v and w must have the same number of components.
$$ \vec{v} \cdot \vec{w} = \begin{pmatrix} 1 \\ 3 \\ 4 \end{pmatrix} \ .* \ \begin{pmatrix} 2 \\ 1 \\ -1 \\ \end{pmatrix} = \begin{pmatrix} 1 \cdot 2 \\ 3 \cdot 1 \\ 4 \cdot -1 \end{pmatrix} = \begin{pmatrix} 2 \\ 3 \\ -4 \end{pmatrix} $$
Scalar-vector multiplication
Multiplying a vector by a scalar uses the same operator as regular multiplication, which is the asterisk *
Where scalar refers to any arbitrary number.
For example, to multiply the scalar 2 by the vector v in Matlab, type 2*v.
>> 2*v
ans =
2
6
8
$$ 2 \cdot \begin{pmatrix} 1 \\ 3 \\ 4 \end{pmatrix} = \begin{pmatrix} 2 \cdot 1 \\ 2 \cdot 3 \\ 2 \cdot 4 \end{pmatrix} = \begin{pmatrix} 2 \\ 6 \\ 8 \end{pmatrix} $$
Scalar-vector multiplication follows the commutative property.
So, you can also write v*2. The result is the same.
>> v*2
ans =
2
6
8
$$ \begin{pmatrix} 1 \\ 3 \\ 4 \end{pmatrix} \cdot 2 = \begin{pmatrix} 1 \cdot 2 \\ 3 \cdot 2 \\ 4 \cdot 2 \end{pmatrix} = \begin{pmatrix} 2 \\ 6 \\ 8 \end{pmatrix} $$
Vector division by a scalar
The division operator between a vector and a scalar is the symbol / (slash).
For example, to divide the vector v by the scalar 2 in Matlab, type v/2
>> v/2
ans =
0.5
1.5
2.0
$$ \frac{ \vec{v} }{2} = \begin{pmatrix} 1 \\ 3 \\ 4 \end{pmatrix} \cdot \frac{1}{2} = \begin{pmatrix} \frac{1}{2} \\ \frac{3}{2} \\ \frac{4}{2} \end{pmatrix} = \begin{pmatrix} 0.5 \\ 1.5 \\ 2 \end{pmatrix} $$
Element-wise Vector Division
Element-wise vector division involves dividing the elements of two vectors that are in the same position.
To perform this type of division, use the symbol ./ (dot and slash)
>> v./w
ans =
0.5
3
-4
Both vectors in element-wise division must be either row vectors or column vectors.
Additionally, the two vectors must have the same number of elements.
$$ \vec{v} \cdot \vec{w} = \begin{pmatrix} 1 \\ 3 \\ 4 \end{pmatrix} \ ./ \ \begin{pmatrix} 2 \\ 1 \\ -1 \end{pmatrix} = \begin{pmatrix} \frac{1}{2} \\ \frac{3}{1} \\ \frac{4}{-1} \end{pmatrix} = \begin{pmatrix} 0.5 \\ 3 \\ -4 \end{pmatrix} $$
Element-wise Vector Exponentiation
In Matlab, you can also perform element-wise exponentiation.
This operation raises the elements of a vector to the same exponent (scalar number).
To perform this operation, use the operator .^
>> v.^2
ans =
1
9
16
$$ \vec{v} \ \text{.^} \ 2 = \begin{pmatrix} 1^2 \\ 3^2 \\ 4^2 \end{pmatrix} = \begin{pmatrix} 1 \\ 9 \\ 16 \end{pmatrix} $$
If the exponent is also a vector, this operation raises each element of the base vector to the element of the exponent vector that is in the same position.
>> v.^w
ans =
1
3
0.25
$$ \vec{v} \ \text{.^} \ \vec{w} = \begin{pmatrix} 1 \\ 3 \\ 4 \end{pmatrix} \ \text{.^} \ \begin{pmatrix} 2 \\ 1 \\ -1 \end{pmatrix} = \begin{pmatrix} 1^2 \\ 3^1 \\ 4^{-1} \end{pmatrix} = \begin{pmatrix} 1 \\ 3 \\ 0.25 \end{pmatrix} $$
In this case, both vectors must be either row vectors or column vectors, and they must have the same number of elements.
Vector Norm (Magnitude or Length)
To calculate the Euclidean norm of a vector, which is the magnitude (length) of the vector, use the norm() function.
>> norm(v)
ans = 5.0990
$$ | \vec{v} | = \sqrt{1^2+3^2+4^2} = \sqrt{1+9+16} = \sqrt{26} = 5,099 $$