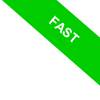
How to calculate derivatives in Matlab
Let me explain to you how to calculate derivatives using Matlab.
So, what exactly is a derivative? Well, a derivative of a function measures the rate at which the function changes at each point in its domain. This is an incredibly useful tool for understanding how a function is behaving, whether it's increasing or decreasing, and even finding the maximum and minimum points of the function.
Derivatives also play a vital role in mathematical analysis, making them a fundamental concept to grasp.
Derivative of a polynomial
To calculate the derivative of a polynomial, you can use the function polyder()
polyder(y)
The parameter y of the function is an array with the numerical coefficients of the polynomial.
Here's a practical example.
Consider this polynomial:
$$ P(x) = 2x^3 + 4x + 3 $$
Define an array with the numerical coefficients of the polynomial ordered by degree.
>> P = [2 0 4 3]
Note. If this last step is not clear, I recommend reading the lesson on how to define a polynomial in Matlab first.
Now, calculate the derivative of the polynomial using the polyder() function:
>> polyder(P)
The result is the first derivative of the polynomial.
ans = 6 0 4
Therefore, the first derivative of the polynomial is:
$$ P'(x) = \frac{d \ P(x)}{dx} = 6x^2 + 4 $$
Verification. The polynomial is composed of the sum of monomials. So to calculate the derivative of the polynomial, you just need to calculate the sum of the first derivatives of the individual monomials. $$ P'(x) = \frac{d \ ( 2x^3 + 4x + 3)}{dx} = \frac{ d \ 2x^3}{dx} + \frac{d \ 4x}{dx} + \frac{d \ 3}{dx} = 6x^2 + 4 + 0 $$
And how do you calculate the second derivative?
To calculate the second derivative of the polynomial, you can iterate the polyder() function multiple times on the result:
>> d1=polyder(P);
>> d2=polyder(d1)
Alternatively, you can create a composite function:
>> polyder(polyder(P))
In both cases, the final result is the second derivative of the polynomial.
ans = 12 0
The second derivative of the polynomial is:
$$ P''(x) = 12x $$
With the same technique, you can calculate the third, fourth, or nth derivative of the polynomial.
Finding the Derivative of a Function
To calculate the derivative of a function with one or more variables, use the diff() function.
diff(function, variable, degree)
The diff() function has three parameters:
- The first parameter is the expression of the function.
- The second parameter is the variable you want to derive (e.g., x, y, etc.).
- The third parameter is the degree of derivation (first derivative, second derivative, third derivative, etc.).
Note. The second and third parameters are optional. If you don't specify the derivation variable in the second parameter, the diff() function defaults to the symbol of the variable x. If you don't specify the degree of derivation, the diff() function calculates the first derivative by default. The diff() function is based on symbolic calculation, so you need to define the variables as symbols through the syms instruction first.
Let me give you a practical example.
Consider the function x3+x2+x with one variable:
$$ f(x) = x^3 + x^2 + x $$
Define the symbol of the variable x:
syms x
To calculate the first derivative of the function, type:
diff(x^3+x^2+x,x,1)
The function has three parameters:
- The first parameter (x^3+x^2+x) is the expression of the function.
- The second parameter (x) is the derivation variable.
- The third parameter (1) is the degree of derivation.
Note. In the function expression, the exponentiation operator is ^.
The diff() function calculates the first derivative of the function with respect to the variable x.
ans =
3*x^2 + 2*x + 1
Therefore, the first derivative of the function is:
$$ f'(x) = 3x^2 +2x+1 $$
Now, let's calculate the second derivative of the same function.
To do this, you just need to indicate 2 in the last parameter of the diff() function:
diff(x^3+x^2+x,x,2)
The result is the second derivative of the function.
ans =
6*x + 2
Therefore, the second derivative of the function is:
$$ f''(x) = 6x +2 $$
Now, let's calculate the third derivative.
Type the same diff() function, but modify the last parameter to 3.
diff(x^3+x^2+x,x,3)
The result is the third derivative of the function.
ans =
6
Therefore, the third derivative of the function is:
$$ f^{(3)}(x) = 6x +2 $$
Partial Derivatives
Matlab allows you to calculate partial derivatives of a function as well.
What is a partial derivative? A partial derivative is a derivative of a function with two or more variables with respect to only one of its variables while holding the other variables constant.
For example, consider this function with two variables:
$$ f(x,y) = x^2 y^2 $$
Define symbols for both independent variables x and y:
syms x y
Now, calculate the first partial derivative of the function x2y2 with respect to the variable x:
diff(x^2*y^2,x,1)
The result is the first partial derivative of the function.
ans
2*x*y^2
Therefore, the first partial derivative of the function with respect to x is:
$$ \frac{\partial \ f(x,y)}{\partial x} = 2xy^2 $$
Now, calculate the first partial derivative of the function x2y2 with respect to the variable y.
diff(x^2*y^2,y,1)
The result is the first partial derivative of the function.
ans
2*x^2*y
Therefore, the first partial derivative of the function with respect to the variable y is
$$ \frac{\partial \ f(x,y)}{ \partial y} = 2x^2y $$
Using this method, you can compute the partial derivatives of any function with two or more variables in Matlab.