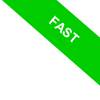
Solving an equation on Matlab
Let me tell you how to solve an equation using Matlab. It's really not too difficult, so let's get started.
There are two different ways you can go about solving an equation on Matlab. The first method involves using polynomials, while the second method is based on symbolic calculation.
The function roots()
Let's start with the roots() function. This function allows you to find the roots of a polynomial.
roots(P)
All you need to do is input the numeric coefficients of the equation as the parameter P.
But what are roots, you ask? They are the values of the unknown variable x that intersect the x-axis and make both sides of the equation equal to zero.
Let me give you a practical example.
Say we have a simple second-degree equation with one unknown variable:
$$ x^2 + 3x = 4 $$
To solve it, we first transform the equation into an equivalent form:
$$ x^2 + 3x -4 = 0 $$
The numeric coefficients of x are 1, 3, and -4, which we then write in an array in descending order of degree: [1 3 -4].
>> P = [1 3 -4];
Note that if one of the coefficients is missing, you should insert a zero in its place.
To find the solutions of the equation, we simply use the roots() function:
>> roots(P)
This will give us the roots of the equation, which in this case are -4 and 1.
ans =
-4
1
To verify the solutions, we can use the polyval() function.
>> polyval(P,roots(P))
ans =
0
0
If we input polyval([1 3 -4], roots([1 3 -4])), we get [0 0], which means that both values of x satisfy the equation.
Verifica. Verifica la prima soluzione. Sostituisci x=-4 nell'equazione. $$ x^2 + 3x -4 = 0 $$ $$ (-4)^2 + 3 \cdot (-4) -4 = 0 $$ $$ 16 -12 -4 = 0 $$ $$ 0 = 0 $$ Ora verifica la seconda soluzione. Sostituisci x=1 nell'equazione. $$ x^2 + 3x -4 = 0 $$ $$ (1)^2 + 3 \cdot 1 -4 = 0 $$ $$ 1 + 3 -4 = 0 $$ $$ 0 = 0 $$ Entrambi i valori risolvono l'equazione.
Now, what if the equation has no solutions in real numbers?
In that case, the roots() function will give us the complex solutions of the equation.
For example, if we have a fourth-degree equation x4+3x2-2x+1=0
$$ x^4 + 3x^2 - 2x +1 = 0 $$
We can use roots([1 0 3 -2 1]) to find the solutions, which turn out to be complex numbers.
>> roots([1 0 3 -2 1])
But don't worry, the roots() function will still give us the correct answers, even if they're not real numbers.
ans =
-0.34975 + 1.74698i
-0.34975 - 1.74698i
0.34975 + 0.43899i
0.34975 - 0.43899i
The solve() function
There's this nifty little function in Matlab called "solve()" that allows you to find the solution of a symbolic equation.
solve(eqz)
It's quite useful, really. All you have to do is pass the symbolic equation you want to solve as a parameter to the solve() function.
Now, when it comes to solving the equation, you need to use symbolic calculation. This involves defining the unknown variables of the problem as symbols, defining the symbolic equation, and then finding the solutions using the solve() function.
Let me give you an example.
Say we have a second-degree equation with one unknown:
$$ x^2 + 3x = 4 $$
To solve this equation using the solve() function, we first need to transform it into normal form by moving all the terms to the left:
$$ x^2 + 3x - 4 = 0 $$
Then we define the unknown variable as a symbolic variable using the "syms" function.
>> syms x
Next, we define the symbolic equation and assign it to the "eqz" variable.
>> eqz = x^2+3*x-4
Finally, we use the solve() function to find the solutions of the equation.
>> solve(eqz)
And there you have it! The solve() function finds the solutions of the equation, which in this case are x1=-4 and x2=1.
ans=
-4
1
It's worth noting that you can use the solve() function and symbolic calculation to solve equations with two or more unknowns.
his makes it a powerful tool for solving equations of any degree in Matlab.