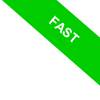
Delete rows or columns of a matrix in Octave
In this lesson I'll explain how to remove a row or a column from a matrix in Octave
I'll give you a practical example
Create a 3x3 square matrix and assign it to variable M
>> M=[1 2 3; 4 5 6; 7 8 9]
M =
1 2 3
4 5 6
7 8 9
The matrix M has three rows and three columns.
Now type the command M(1,:)=[ ] to delete the first row of the matrix
>> M(1,:)=[ ]
M =
4 5 6
7 8 9
How it works?
- The number 1 in the first parameter M(1, :) selects the first row of the matrix M
- The colon character (:) in the second parameter M(1, :) selects all columns
The command M(1,:)=[ ] selects all the columns of the first row and assigns them a null value [].
$$ \begin{pmatrix} \not{1} & \not{2} & \not{3} \\ 4 & 5 & 6 \\ 7 & 8 & 9 \end{pmatrix} $$
The first row of the matrix M is deleted.
The final result is a 2x3 matrix with two rows and three columns.
$$ \begin{pmatrix} 4 & 5 & 6 \\ 7 & 8 & 9 \end{pmatrix} $$
Note. To delete the other lines, type the number of the line you want to remove. For example, to delete the second row of the matrix type M(2,:)=[ ]. The index number of the first row is 1, that of the second row is 2, and so on.
I'll give you another practical example
Make the 3x3 matrix again
>> M=[1 2 3; 4 5 6; 7 8 9]
M =
1 2 3
4 5 6
7 8 9
Now type M(:, 3)=[ ] to delete the third column from the matrix
>> M(:,3)=[ ]
M =
1 2
4 5
7 8
The command M(:, 3)=[ ] selects all rows (:) of the third column of the matrix and assigns them a null value [ ].
$$ \begin{pmatrix} 1 & 2 & \not{3} \\ 4 & 5 & \not{6} \\ 7 & 8 & \not{9} \end{pmatrix} $$
The third column is dropped from the matrix.
$$ \begin{pmatrix} 1 & 2 \\ 4 & 5 \\ 7 & 8 \end{pmatrix} $$
The final result is a 3x2 matrix with three rows and two columns.
How do you delete multiple rows or columns?
When you need to remove multiple rows or columns from the matrix, type the list of columns or rows you want to delete in square brackets
For example, make a 4x4 square matrix with four rows and four columns.
>> M=[1 2 3 4; 5 6 7 8; 9 8 7 6; 5 4 3 2]
M =
1 2 3 4
5 6 7 8
9 8 7 6
5 4 3 2
Now type the command M([1 4],:)=[ ] to delete the first row and the last row of the matrix.
Type the list of rows to be deleted in square brackets [1 4] in the first parameter of the command, separating the numbers of the lines from each other with a space or a comma.
>> M([1 4] ,:)=[ ]
M =
5 6 7 8
9 8 7 6
This command deletes the first and third rows of the matrix
$$ \begin{pmatrix} \not{1} & \not{2} & \not{3} & \not{4} \\ 5 & 6 & 7 & 8 \\ 9 & 8 & 7 & 6 \\ \not{5} & \not{4} & \not{3} & \not{2} \end{pmatrix} $$
The result is a 2x4 matrix
$$ \begin{pmatrix} 5 & 6 & 7 & 8 \\ 9 & 8 & 7 & 6 \end{pmatrix} $$
Now type the command M(:,[2 3 4])=[ ] to delete the second, third and fourth column of the matrix.
In this case the list of columns to be deleted [2 3 4] consists of three columns
>> M(:,[2 3 4])=[ ]
M =
5
9
This command deletes the second, third and fourth columns of the matrix.
$$ \begin{pmatrix} 5 & \not{6} & \not{7} & \not{6} \\ 9 & \not{8} & \not{7} & \not{6} \end{pmatrix} $$
The final result is a 2x1 matrix that is a column vector
$$ \begin{pmatrix} 5 \\ 6 \end{pmatrix} $$
I conclude this lesson with some practical advice.
When the rows or columns are continuous, it is better to indicate the interval between the first and the last, inserting a colon as a separator (:).
For example, type M(:,[2: 4])=[ ] to delete the second, third and fourth columns of the matrix M.
This way you are indicating the interval between columns [2: 4] instead of the list [2 3 4]
>> M(:,[2:4])=[ ]
M =
5
9
The end result is always the same. The columns are removed from the matrix