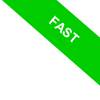
How to change the number of rows and columns of a matrix in Octave
In this lesson I'll explain how to change the number of rows and columns of a matrix in Octave without changing the total number of elements.
I'll give you a practical example.
Create a 3x2 rectangular matrix with three rows and two columns.
>> M = [ 1 2 ; 3 4 ; 5 6 ]
M =
1 2
3 4
5 6
Now transform the 3x2 matrix into a 2x3 matrix with two rows and three columns.
Type reshape(M,2,3)
>> reshape(M,2,3)
ans =
1 5 4
3 2 6
You can also turn the initial matrix into a row vector by typing reshape(M,1,6)
>> reshape(M,1,6)
ans =
1 3 5 2 4 6
or in a column vector by typing reshape(M,6,1)
>> reshape(M,6,1)
ans =
1
3
5
2
4
6
In all cases the number of elements is unchanged.
Note. A vector is a particular matrix with a single 1x6 row or a single 6x1 column.
Similarly you can transform a vector into a matrix.
For example, create a vector with eight elements
>> v = [ 1 2 3 4 5 6 7 8 ]
v =
1 2 3 4 5 6 7 8
Transform the vector into a 2x4 matrix with two rows and four columns by typing reshape(v,2,4)
>> reshape(v, 2, 4)
ans =
1 3 5 7
2 4 6 8
Alternatively, you can turn the vector into a 4x2 matrix by typing reshape(v, 4, 2)
>> reshape(v, 4, 2)
ans =
1 5
2 6
3 7
4 8
This way you can convert a vector to a matrix or vice versa as long as the number of elements remains the same.
If the number of elements is different, the reshape() command fails.
Ad esempio, non puoi trasformare una matrice 4x2 in una matrice 3x3 perché la prima matrice ha otto elementi mentre la seconda matrice ha nove elementi.
In this case the reshape() command fails.
>> reshape(M,3,3)
error: reshape: can't reshape 3x2 array to 3x3 array