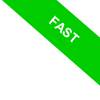
How to replace the diagonals of a matrix in Octave
In this lesson I'll show you how to replace the elements in a diagonal of the matrix
What are the diagonals of a matrix? They are the elements on the diagonals that start at the top right and end at the bottom left and vice versa. For example, the main diagonal of the matrix M consists of the elements 1, 5, 9.$$ M = \begin{pmatrix} \color{red}1 & 2 & 3 \\ 4 & \color{red}5 & 6 \\ 7 & 8 & \color{red}9 \end{pmatrix} $$
I'll give you a practical example.
Create a 3x3 matrix
>> M=[1 2 3 ; 4 5 6 ; 7 8 9]
M =
1 2 3
4 5 6
7 8 9
The main diagonal consists of the elements 1, 5, 9.
$$ M = \begin{pmatrix} \color{red}1 & 2 & 3 \\ 4 & \color{red}5 & 6 \\ 7 & 8 & \color{red}9 \end{pmatrix} $$
To replace the main diagonal elements with -1, -2, -3, type spdiags([-1;-5;-9],0,M)
>> spdiags([-1;-5;-9],0,M)
ans =
-1 2 3
4 -5 6
7 8 -9
- The first parameter is a column vector [-1;-5;-9] with the new diagonal elements.
- The second parameter (0) is the index of the diagonal. The main diagonal has index 0.
Note. Index 1 is the diagonal above the main diagonal while index -1 is the diagonal below.
- The third parameter M is the variable where the matrix is stored.
The output result is a square matrix with the new elements in the main diagonal.
$$ M = \begin{pmatrix} \color{red}{-1} & 2 & 3 \\ 4 & \color{red}{-5} & 6 \\ 7 & 8 & \color{red}{-9} \end{pmatrix} $$
To change the elements on the diagonal above the main diagonal, type spdiags([-1;-5;-9],1,M)
>> spdiags([-1;-5;-9],1,M)
ans =
1 -5 3
4 5 -9
7 8 9
The function replaces the elements above the main diagonal.
$$ M = \begin{pmatrix} 1 & \color{red}{-5} & 3 \\ 4 & 5 & \color{red}{-9} \\ 7 & 8 & 9 \end{pmatrix} $$
Note. The first element -1 of the column vector [-1;-5;-9] does not appear in the new matrix because, in this case, it is outside the matrix.
To replace elements on the secondary diagonal combine functions spdiags() and fliplr()
>> fliplr(spdiags([-1;-5;-9],0,fliplr(M)))
ans =
1 2 -1
4 -5 6
-9 8 9
The function replaces the values on the secondary diagonal of the matrix.
The secondary diagonal starts at the top right and ends at the bottom left.
$$ M = \begin{pmatrix} 1 & 2 & \color{red}{-1} \\ 4 & \color{red}{-5} & 6 \\ \color{red}{-9} & 8 & 9 \end{pmatrix} $$
This way you can replace all values on the secondary diagonals of the matrix as well.