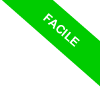
The Adjugate Matrix in Octave
In this tutorial, we're going to delve into the process of calculating the adjugate matrix using Octave.
First off, let's clarify what an adjugate matrix is. Simply put, it's the transpose of the cofactor matrix derived from a square matrix.
To compute the adjugate matrix, you'll need to multiply the inverse of the original matrix by its determinant, like so:
>> inv(A)*det(A)
Let's put this into practice. Start by defining a 3x3 square matrix, assigning it to the variable A:
>> A=[1 2 0 ; 3 4 5; 0 1 1]
Next, calculate its adjugate matrix using the previously mentioned expression:
>> inv(A)*det(A)
ans =
-1 -2 10
-3 1 -5
3 -1 -2
In this way, you obtain the adjugate matrix of A.
Alternatively, the adjugate matrix can be computed using the adjoint() function of symbolic package.
adjoint()
The symbolic package in Octave offers a host of additional features for symbolic manipulation of mathematical expressions.
If you haven't installed it yet, you can do so with the following command:
pkg install -forge symbolic
If you've previously installed it, simply load the symbolic package into memory:
pkg load symbolic
Once the symbolic package is loaded, you can use the adjoint() function to calculate the adjugate matrix.
Here's a hands-on example.
Define a square matrix in the variable A:
>> A=[1 2 0 ; 3 4 5; 0 1 1]
Then, use the adjoint(A) function to calculate the adjugate matrix, storing the result in the variable adj_A
>> adj_A = adjoint(A);
The adjugate matrix is now stored in the variable adj_A
>> adj_A
adj_A =
-1 -2 10
-3 1 -5
3 -1 -2
If you encounter any issues, there's an alternative method that utilizes symbolic computation.
Convert the matrix into a symbolic object with the sym command
A_sym = sym(A);
Then, calculate the adjugate matrix of A using the adjoint() function
adj_A = adjoint(A_sym);
Finally, convert the result back into a numeric matrix.
adj_A_num = double(adj_A)
The final result is the adjugate matrix of A
>> adj_A
adj_A = (sym 3×3 matrix)
-1 -2 10
-3 1 -5
3 -1 -2
It's important to note that the adjugate matrix is only defined for square matrices.
Attempting to calculate the adjugate matrix of a non-square matrix will result in an error in Octave.