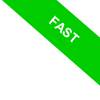
Sparse matrix in Octave
In this online lesson I'll explain how to make a sparse matrix on Octave.
What is a sparse matrix? What is it for? When you create a very large matrix with many zeros you are unnecessarily occupying a large part of the computer's memory. For example, a diagonal matrix has nonzero values only on the leading diagonal and zeros elsewhere. $$ M = \begin{pmatrix} 1 & 0 & 0 & 0 \\ 0 & 1 & 0 & 0 \\ 0 & 0 & 1 & 0 \\ 0 & 0 & 0 & 1 \\ \end{pmatrix} $$ You can achieve the same result by creating a sparse matrix where the zeros are compressed. This way you take up less memory.
You can use sparse matrices to create identity matrices and compressed diagonal matrices.
The sparse identity matrix
I'll give you a practical example
Type eye(4) to create an identity matrix
>> eye(4)
ans =
Diagonal Matrix1 0 0 0
0 1 0 0
0 0 1 0
0 0 0 1
There are 12 zeros and 4 ones in the matrix. Much of the space is unnecessarily occupied by null values.
You can make the same identity matrix using the sparse matrix technique using the speye(4) command
>> speye(4)
ans =
Compressed Column Sparse (rows = 4, cols = 4, nnz = 4 [25%])
(1, 1) -> 1
(2, 2) -> 1
(3, 3) -> 1
(4, 4) -> 1
The sparse matrix only stores the positions (row, column) where there are non-zero values, taking up less memory space.
For this reason it is called a "sparse" matrix.
Note. Only useful information is stored in the sparse matrix. In an identity matrix, null values are irrelevant. Therefore they are eliminated. This reduces the memory space occupied and makes the matrix calculation faster. Particularly if the matrix is very large.
You can use the sparse matrix you just created in your calculations as well as if it were a 4x4 identity matrix
For example, create a matrix with 4 columns
>> M=[1 2 3 4;5 6 7 8; 9 0 1 2; 3 4 5 6]
M =
1 2 3 4
5 6 7 8
9 0 1 2
3 4 5 6
Now multiply matrix M by the identity matrix
>> M*eye(4)
ans =
1 2 3 4
5 6 7 8
9 0 1 2
3 4 5 6
Then multiply the M matrix by the sparse identity matrix
>> M*speye(4)
ans =
1 2 3 4
5 6 7 8
9 0 1 2
3 4 5 6
The result is the same.
In the second case you have occupied less memory and the computation time is less.
The Sparse Diagonal Matrix
Use spdiags() to create a sparse diagonal matrix with values other than 1 uses
>> spdiags([1;2;3],0,3,3)
ans =
Compressed Column Sparse (rows = 3, cols = 3, nnz = 3 [33%])
(1, 1) -> 1
(2, 2) -> 2
(3, 3) -> 3
- The first parameter is a column vector [1; 2; 3] with the elements of the diagonal
- the second parameter is the index number of the diagonal, where the number zero (0) is the main diagonal
- the third and fourth parameters are the number of rows (3) and columns (3) of the sparse matrix
The output result is the sparse matrix of a 3x3 diagonal matrix
$$ M = \begin{pmatrix} 1 & 0 & 0 \\ 0 & 2 & 0 \\ 0 & 0 & 3 \\ \end{pmatrix} $$
Define a sparse matrix by indicating only the non-zero values
You can also create a sparse matrix by specifying only non-null values and their position in the matrix.
For example, create a sparse matrix with these values
$$ M = \begin{pmatrix} 3 & 0 & 1 \\ 0 & 1 & 2 \\ 4 & 0 & 0 \end{pmatrix} $$
Define an array with as many rows as there are non-null values of the array.
Write the row number, column number, and non-null element value in each row of the array.
>> v = [1 1 3; 1 3 1; 2 2 1; 2 3 2; 3 1 4]
v =
1 1 3
1 3 1
2 2 1
2 3 2
3 1 4
Note. The first non-null element (3) is in the first row and column (1,1). Therefore, the first row of the vector is 1 1 3. The second non-null element (1) is in the first row in the third column. Hence, the second row of the vector is 1 3 1. The third non-null element (2) is in the second row in the second column. So, the third row of the vector is 2 2 2. And so on.
Now to create the sparse matrix use spconvert() function
>> spconvert(v)
The result is a sparse matrix with the values in the positions you indicated.
ans =
Compressed Column Sparse (rows = 3, cols = 3, nnz = 5 [56%])
(1, 1) -> 3
(3, 1) -> 4
(2, 2) -> 1
(1, 3) -> 1
(2, 3) -> 2
Nell'array usato per costruire la matrice sparsa puoi definire i valori non nulli seguendo qualsiasi ordine.
Note. You can also indicate multiple values for the same position in the matrix. When a position in the array is present multiple times in the array, Octave adds the values together without going into error. For example, define a sparse matrix by indicating in position (1,1) the value 2 and the value 3. In position (1,1) Octave adds the two values 2 + 3 = 5
With this method you can also create a sparse matrix with complex numbers.