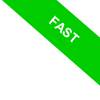
How to calculate the inverse matrix in Octave
In this lesson I'll explain how to calculate the inverse matrix of a square or rectangular matrix in Octave.
What is the inverse matrix? A matrix M is invertible if there is an inverse matrix M-1 such that the product between the two matrices is an identity matrix. The identity matrix is a matrix with 1 on the main diagonal and 0 on all other elements. For example $$ M \cdot M^{-1} = I $$
I'll give you a practical example
Define a 2x2 square matrix
>> M=[1 2;3 4]
M =
1 2
3 4
To calculate the inverse matrix of M use the inv() function
>> inv(M)
ans =
-2.00000 1.00000
1.50000 -0.50000
Now multiply the matrix M with its inverse inv (M)
The result is an identity matrix
>> M*inv(M)
ans =
1.00000 0.00000
0.00000 1.00000
You can use the inv() function only if the matrix M is a square matrix.
Note. Not all square matrices are invertible. For example, there is no inverse matrix of square matrices with null determinant (called singular matrices). When there is no inverse matrix Octave displays a warning message "Matrix is singular to working precision". To avoid making a mistake, I always recommend that you verify that the product M * inv (M) is an identity matrix.
How to calculate the inverse matrix in rectangular matrices?
In Octave you can also calculate the inverse matrix of a rectangular matrix.
In this case, however, you have to use the pseudo inverse function pinv()
For example, define a 2x3 rectangular matrix with two rows and three columns
>> M2=[1 2 3 ; 4 5 6]
M2 =
1 2 3
4 5 6
Now calculate the inverse matrix of the rectangular matrix using the pinv() function
>> pinv(M2)
ans =
-0.94444 0.44444
-0.11111 0.11111
0.72222 -0.22222
Multiply the rectangular matrix M2 by its inverse (M2). The result is an identity matrix.
>> M2*pinv(M2)
ans =
1.00000 -0.00000
0.00000 1.00000
This way you can find inverse matrices even of rectangular matrices.
Note. You can also use the pinv() function to calculate the inverse matrix of a square matrix instead of the inv() function. The result is always the same. You cannot use the inv() function on rectangular matrices.