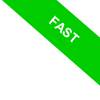
Indentation in the Python language
In this lesson, I will explain everything you need to know about indentation in Python.
What is indentation in Python? Indentation is the way code is structured in a program written in the Python language. Most programming languages use curly braces to delimit nested code blocks. In the Python language, however, to write a nested code block, you need to indent it to the right by a few white spaces.
In practice, when you write a program in Python, all code blocks must be indented to the right by the same number of spaces.
Additionally, before every nested code block, there must always be a statement that ends with the colon character:
Let me give you a practical example.
This simple script consists of a conditional structure:
- if x > 5:
- print("x is greater than 5")
- else:
- print("x is less than or equal to 5")
The if-else conditional structure has two blocks:
- If the value of x is greater than 5, the script writes "x is greater than 5" (first block).
- Otherwise, it writes "x is less than or equal to 5" (second block).
As you can see, the two blocks are indented a few spaces more to the right than the if and else statements that contain them.
Note. In other programming languages, parentheses are used to group blocks. For example, in the C language, curly braces are used.
if (x>5) {
printf("the number is positive");
} else {
printf("the number is negative");
}
What is the difference between C and Python? In the C language, indentation is optional and curly braces are mandatory. On the other hand, in Python, indentation is mandatory, and parentheses are optional to delimit a code block.
What is the purpose of indentation?
Indentation helps make the code more readable, clean, and easy to understand. It makes it well-structured.
Furthermore, it is not just a matter of style because in Python, indentation is mandatory.
If you do not correctly follow the indentation, the program will not work, and the Python interpreter will return an error message.
- def print_numbers(n):
- for i in range(n):
- if i % 2 == 0:
- print(i, "is even")
- else:
- print(i, "is odd")
Therefore, indentation is very important in Python because it is an integral part of the syntax of the programming language.
How many white spaces should you use for code indentation?
There is no predefined number. You can indent the text to the right by only one character or more characters.
However, you must maintain the same number of spaces within the code block. Once chosen, you cannot change it.
Note. Generally, the default value for indentation in Python is 4 spaces, but you can choose any number of spaces you prefer. However, I recommend not using spaces and tabs together because they are not the same thing. For example, if you started a block with an indent of 5 white spaces, you must continue to write the block with an indent of 5 white spaces. Neither more nor less. Any other nested blocks inside must be indented with more than 5 white spaces, and so on.
This guarantees that the program works correctly and that the statements are executed in the correct order.
In conclusion, indentation in Python is a fundamental concept for programming in Python.
In addition to making the code structured and easy to read, it is an essential condition for the proper functioning of the program.