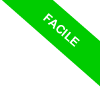
Ordered Dictionaries in Python
An ordered dictionary in Python is more than just a collection; it's a way to keep your data structured while preserving the sequence of insertion. Essentially, it's an instance of the OrderedDict class, an enhanced version of the standard dictionary.
OrderedDict()
What sets it apart is its ability to not only store key-value pairs like any dictionary but to also remember the exact sequence in which each entry was added.
With an ordered dictionary, you can ensure that your data is displayed in the same order you added it, every time.
Why Use an Ordered Dictionary? In the world of Python programming, the order of elements can be just as important as the elements themselves. Standard dictionaries make no guarantees about maintaining this order. However, with ordered dictionaries, you have a reliable way to keep your data sequence intact. This feature has been a key component of Python since version 3.7.
Let's delve into ordered dictionaries with a real-world example.
Consider a shopping list, where the order of items matters because it reflects your shopping priorities or the sequence in which you plan to buy them.
- 5 apples
- 2 loaves of bread
- 1 carton of milk
To represent this list in Python while keeping the order of items, an OrderedDict is your go-to choice.
Start by importing OrderedDict from the collections module.
from collections import OrderedDict
Creating an OrderedDict is as straightforward as setting up a standard dictionary.
shopping_list = OrderedDict()
Once you've set it up, adding items is a breeze. Let's populate your shopping list.
shopping_list['apples'] = 5
shopping_list['bread'] = 2
shopping_list['milk'] = 1
The beauty of OrderedDict is that it preserves the order: apples, bread, and milk are stored just as you've entered them.
Iterating over the dictionary retains this order, showcasing Python's attention to your specified sequence.
- for item, quantity in shopping_list.items():
- print(f"{item}: {quantity}")
Your items are listed exactly in the order you added them.
apples: 5
bread: 2
milk: 1
Need to Reorder?
Changing the order in an OrderedDict isn't an ordeal. To rearrange items, simply remove and reinsert them.
Let's say you want to move apples to the end of the list. Start by removing it:
del shopping_list['apples']
With apples gone, your list temporarily shrinks to two items.
bread: 2
milk: 1
Reintroduce apples, and it takes its new place at the end of the list.
shopping_list['apples'] = 5
And just like that, apples are back, this time at the bottom.
bread: 2
milk: 1
apples: 5
Alternatively, OrderedDict's move_to_end() method offers a slick way to shift items to either end of the dictionary without removing them.
This method is exclusive to ordered dictionaries, adding to their utility.
shopping_list.move_to_end('apples')
Keep in mind that for two OrderedDicts to be considered equal, they must match in both content and order, a distinct feature from regular dictionaries.
Besides all standard dictionary methods, an ordered dictionary boasts unique functions, enhancing its versatility.
For example, the popitem() method in an ordered dictionary smartly returns and removes an item based on its position, either the last or the first.
shopping_list.popitem()
In a standard dictionary, however, the popitem() method returns and removes a key-value pair.
In essence, OrderedDict shines when the order of elements is paramount, offering a clear and explicit way to maintain sequence in your data collections.