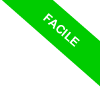
Python's move_to_end() Method in OrderedDict
The OrderedDict.move_to_end() method in Python is a powerful tool for repositioning an item to either the beginning or end of an ordered dictionary. Exclusive to the ordered dictionaries crafted with the OrderedDict class, this method is not applicable to standard dictionaries.
OrderedDict.move_to_end(key, last=True)
Functionality of move_to_end() includes:
- key: Identifies the item to be moved.
- last: Determines the item's new position. Set to True for moving it to the end, or False for the beginning. By default, it's set to True.
Essentially, the move_to_end() method relocates a specific key within the ordered dictionary, positioning it at either the forefront or the tail end of the OrderedDict.
Understanding OrderedDict is crucial before delving into the specifics of move_to_end().
What Exactly is OrderedDict? A specialized subclass of the standard dictionary, OrderedDict retains the order of item insertion. Unlike standard dictionaries in Python that lack an inherent order, OrderedDict preserves the sequence of entered keys. While Python 3.7 and later versions inherently maintain insertion order, earlier versions or scenarios requiring a guaranteed sequence rely on OrderedDict.
To better grasp its application, let's explore a hands-on example.
Begin by importing the OrderedDict class.
from collections import OrderedDict
Next, create an ordered dictionary using OrderedDict, populating it with a few key-value pairs.
orders = OrderedDict([('pizza', 2), ('soda', 5), ('sandwich', 3)])
Now, reposition 'soda' to the dictionary's end with the move_to_end() method.
orders.move_to_end('soda')
Examine the dictionary to observe the shift.
print(orders)
Notice the altered sequence, with 'soda' now at the end.
OrderedDict([('pizza', 2), ('sandwich', 3), ('soda', 5)])
What if We Want to Move an Item to the Start?
Employing the same method, you can easily shift an item to the dictionary's beginning.
For example, move 'sandwich' to the start by setting last=False.
orders.move_to_end('sandwich', last=False)
Review the dictionary again to see the change.
print(orders)
'Sandwich' now leads the dictionary's lineup.
OrderedDict([('sandwich', 3), ('pizza', 2), ('soda', 5)])
Optimal Use Cases for move_to_end()
This method is particularly effective in creating stack-like structures, queues, or LRU (Least Recently Used) caches, where the position of recently accessed items is critical. It's also ideal for sorting items by specific criteria, such as usage frequency or priority, or in scenarios where the order of processing is a key factor.