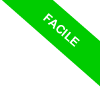
Python's Dictionary Comprehension
Welcome to our comprehensive guide on utilizing Python's dictionary comprehension—an incredibly powerful tool that's often not given enough credit. Let's dive in!
What is it good for? Picture this: you have a list of words and your task is to pair each word with its length in a dictionary. Instead of resorting to a lengthy and monotonous loop, dictionary comprehension streamlines the process into a single, elegant line of code.
Let’s start with a basic example. Say you have a list of words:
words = ["apple", "banana", "watermelon"]
With Dictionary Comprehension, you can effortlessly create a dictionary linking each word to its length, using a for loop.
lengths = {word: len(word) for word in words}
In this code, the variable "word" cycles through each item in the words list. Simultaneously, the len() function determines the length of each word.
Now, let’s see the result. Print the dictionary:
print(lengths)
Voilà! Each word from our list is now a key in the dictionary, with its corresponding value being the length of the word.
{'apple': 5, 'banana': 6, 'watermelon': 10}
Dictionary comprehension’s versatility doesn’t stop there. You can add conditions to tailor your dictionary further. For instance, if your goal is to include only words exceeding 5 letters, simply tweak the code like so:
lengths = {word: len(word) for word in words if len(word) > 5}
This modification ensures that the dictionary includes only words with more than five characters.
{'banana': 6, 'watermelon': 10}
Notice how "apple" is now excluded, as it contains just 5 letters.
To wrap up, dictionary comprehension is not just a time-saver but a refined method for transforming a list, or any collection for that matter, into a dictionary. It’s a testament to Python’s ability to facilitate clean and efficient coding.
Invest some time in practicing this technique, and it's sure to become a staple in your Python toolkit.