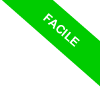
Python's update() Method
Python has a compelling feature, the update() method, which provides the functionality to rejuvenate your dictionary with elements derived from another dictionary.
This is how you employ it:
dictionary1.update(dictionary2)
Through this operation, the items from your second dictionary are seamlessly integrated into your first dictionary. It's a merging of sorts.
However, if a key from the first dictionary mirrors a key in the second, the update() method will only refresh the corresponding value from the first dictionary.
An essential caveat to remember is that Python will only consider the last occurrence of any repeated items present in the second dictionary that you're passing to the update() method.
Let's bring this concept to life with a hands-on example.
Imagine that we have a dictionary assigned to a variable "dict1", housing two keys: "a" and "b".
dict1 = {'a': 1, 'b': 2}
We then create a second dictionary, "dict2", containing two other keys: "b" and "c".
dict2 = {'b': 3, 'c': 4}
The stage is set. We now engage the update() method to infuse "dict1" with the elements present in "dict2".
dict1.update(dict2)
As the final act, we print the content of the revitalized "dict1" dictionary.
print(dict1)
Post operation, "dict1" accommodates three keys: "a", "b", and "c".
{'a': 1, 'b': 3, 'c': 4}
In reviewing the outcome, it's evident that the value of 'b' in dict1 has been superseded by the 'b' value from dict2, and additionally, the new key-value pair 'c': 4 has been assimilated into dict1.