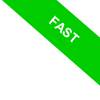
Python's keys() Method
Let's delve into Python's keys() method, a useful function which provides a lens into the key objects of a dictionary.
The syntax is as simple as:
object.keys()
To illustrate, consider the following real-world example.
Suppose you're managing an inventory system and you've got a dictionary named "store". The dictionary might look something like this:
store = { "apples": 50, "bananas": 25, "oranges": 33, "kiwis": 10, "cherries": 15, }
When the time comes to glean a list of all keys in this dictionary, you'd employ the keys() method in this way:
k = store.keys()
print(k)
Executing this code yields all the keys of the dictionary.
The output would resemble:
dict_keys(['apples', 'bananas', 'oranges', 'kiwis', 'cherries'])
Take note, though, that the resulting output isn't a standard list, but a dict_keys object.
For instances when you need a proper list, the solution is straightforward. You can swiftly convert it, using the following code:
k= list(store.keys())
print(k)
Now, the same key data appears as a list object.
['apples', 'bananas', 'oranges', 'kiwis', 'cherries']
In this case, 'k' becomes a standard Python list housing the dictionary keys.
Alternatively, suppose you're in a scenario where you'd like to read the keys in an iterative manner.
You could construct a for loop to cycle through the keys from the dict_keys object:
- for i in store.keys():
- print(i)
Or, you could even get the keys directly from the dictionary itself:
- for i in store:
- print(i)
Both these strategies will churn out a similar outcome, presenting a list of keys.
apples
bananas
oranges
kiwis
cherries
To summarize, the keys() method is an invaluable tool when working with dictionaries in Python, providing you with flexibility and control in managing and accessing your data.