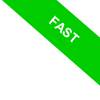
Python's popitem() Method
Python's dictionary class houses a built-in method known as popitem().
Here's the basic structure:
object.popitem()
The primary function of popitem() is to extract and return a key-value pair, formatted as a tuple, from the dictionary.
The item selected for removal is always the one most recently added.
However, one should be aware that if the method is called on an empty dictionary, it triggers a KeyError exception.
Let's dive into a practical demonstration of its application.
Imagine we have a dictionary named "city_population", populated with the following key-value pairs.
city_population = {
'New York': 8623000,
'Los Angeles': 3999000,
'Chicago': 2716000,
'Houston': 2313000,
'Phoenix': 1660000
}
The next step involves invoking the popitem() method to extract the last key from the dictionary.
key, value = city_population.popitem()
What the method accomplishes here is to take the most recent key and its corresponding value, unpack them, and subsequently assign them to the "key" and "value" variables.
After this, we can inspect the content of these variables:
print(f"The last inserted city was {key} with a population of {value}.")
With this execution, the method has extracted the last key, "Phoenix", along with its associated value of 1660000, leading to the output.
The last inserted city was Phoenix with a population of 1660000.
In this instance, popitem() has successfully removed the last inserted item, ('Phoenix': 1660000), from the dictionary, returning it in the form of a tuple.
To verify this change, we can examine the content of the "city_population" dictionary
print( city_population.items() )
As expected, the key "Phoenix" is conspicuously absent from the dictionary.
dict_items([('New York', 8623000), ('Los Angeles', 3999000), ('Chicago', 2716000), ('Houston', 2313000)])
This example effectively demonstrates how the popitem() method serves a dual purpose, enabling the extraction and simultaneous removal of any key from a Python dictionary.