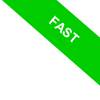
Python's copy() Method
In the realm of Python programming, the copy() method serves as a convenient tool for generating a replica of an object - be it a list, dictionary or more. Simply put, you'd use:
object.copy()
In this expression, "object" signifies the variable which holds the actual object, which could potentially be a list, a dictionary, or any other similar data structure.
This feature is particularly beneficial when your task requires manipulating a copy, whilst ensuring the original object remains untouched.
The Versatility of the copy() Method with Lists
To illustrate its usage, let's consider a practical example.
First, we define a list inside the variable named "list":
list = [1, 2, 3, 4]
Then, we go on to produce a duplicate of this list by deploying the copy() method, and assign it to a new variable "list_copy":
list_copy = list.copy()
Here, Python handily crafts an exact replica of the original list within our "list_copy" variable.
Suppose we append an element to the list_copy via the append() method:
list_copy.append(5)
This action modifies "list_copy", but importantly, the original "list" remains intact.
When printing the content of the original list, it stays consistent with its initial four elements:
print(lista)
[1, 2, 3, 4]
On the flip side, printing the contents of "list_copy" reveals it now houses five elements:
print(lista_copy)
[1, 2, 3, 4, 5]
It's crucial, however, to note that Python's copy() method performs what's known as a 'shallow copy'.
This implies that if your original object contains references to other objects (for instance, a list of lists), the copy() method duplicates only the references, leaving the referred objects themselves untouched.
Consider a list of lists.
list = [[1, 2, 3], [4, 5, 6]]
Then we apply the copy() method to duplicate this list:
list_copy = list.copy()
Here, the copy() method confines its operations to duplicating references to the internal structures, not the individual values within them.
Let's modify an element of the "list_copy" variable:
list_copy[0][0] = 9
Interestingly, this change impacts both the "list_copy" and the original list:
print(lista_copy)
[[9, 2, 3], [4, 5, 6]]
print(list)
[[9, 2, 3], [4, 5, 6]]
To create a 'deep copy' that also duplicates the internal objects within the list, you'll need to utilise the deepcopy() function from the copy module.
Let's recreate our list of lists:
list = [[1, 2, 3], [4, 5, 6]]
First, import the copy module to get access to all its functions:
import copy
Then we execute a deep copy of the list:
lista_copy = copy.deepcopy(list)
Now, let's tweak an element within the copied list:
lista_copia[0][0] = 9
In this scenario, adjusting an internal element of "list_copy" leaves the corresponding element in the original list unaltered:
print(list_copy)
[[9, 2, 3], [4, 5, 6]]
print(list)
[[1, 2, 3], [4, 5, 6]]
Understanding Dictionary Copy in Python
Utilizing the copy method on a Python dictionary results in a shallow copy of the original dictionary. This means it duplicates the dictionary structure, but not the elements contained within.
Consider this scenario: you define a dictionary:
original_dictionary = {'name': 'Mario', 'age': 30, 'city': 'Rome'}
To duplicate this dictionary, you employ the copy method:
dictionary_copy = original_dictionary.copy()
Suppose you then alter an element in the duplicate dictionary:
dictionary_copy['age'] = 35
When you print both dictionaries, the difference becomes apparent. Starting with the original:
print("Original Dictionary:", original_dictionary)
Original Dictionary: {'name': 'Mario', 'age': 30, 'city': 'Rome'}
Followed by the duplicate:
print("Duplicate Dictionary:", dictionary_copy)
Duplicate Dictionary: {'name': 'Mario', 'age': 35, 'city': 'Rome'}
This illustrates that altering the duplicate does not impact the original dictionary, showcasing their independence.
However, this principle of independence applies only to shallow copies. With nested objects within the dictionary, such as another dictionary or a list, both the original and the duplicate reference the same nested object.
For instance, if you have a nested dictionary:
original_dictionary = {
'name': 'Mario',
'age': 30,
'address': {
'street': 'Via Roma',
'number': 10
}
}
Creating a shallow copy:
dictionary_copy = original_dictionary.copy()
And modifying a nested object:
dictionary_copy['address']['street'] = 'Via Milano'
Will reflect in both dictionaries. Observe the original dictionary:
print("Original Dictionary:", original_dictionary)
Original Dictionary: {'name': 'Mario', 'age': 30, 'address': {'street': 'Via Milano', 'number': 10}}
And the duplicate:
print("Duplicate Dictionary:", dictionary_copy)
Duplicate Dictionary: {'name': 'Mario', 'age': 30, 'address': {'street': 'Via Milano', 'number': 10}}
This phenomenon occurs because a shallow copy merely replicates the reference to the nested object, not the object itself. To create completely independent copies, including the nested objects, Python’s 'copy' module provides a 'deepcopy' method.