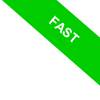
Python's fromkeys Method
The fromkeys() method in Python is a versatile tool for creating a dictionary by specifying keys and assigning a single, common value to all of them.
dict.fromkeys(seq[, value])
This static method belongs to the dict class and requires two parameters:
- seq represents the sequence of keys for the new dictionary.
- value is the uniform value assigned to each key. This parameter is optional and defaults to None if left unspecified.
When you need to initialize a dictionary with a predefined set of keys and a uniform value, fromkeys() is exceptionally useful.
Essentially, every key will share the identical value or reference.
Practical Applications: Ideal for setting up dictionaries for data aggregation or counting purposes. It's also great for quickly generating dictionaries with preset values for testing or prototyping scenarios.
Let's dive into a practical example:
Start by defining a list of keys.
keys = ['x', 'y', 'z']
Next, use fromkeys to generate a dictionary from these keys.
dictionary = dict.fromkeys(keys)
Printing the dictionary reveals:
print(dictionary)
Without specifying a value, each dictionary key defaults to None.
{'x': None, 'y': None, 'z': None}
Now, for another example:
Using the same key list:
keys = ['x', 'y', 'z']
Create another dictionary, this time assigning a value of 1 to each key.
dictionary2 = dict.fromkeys(keys, 1)
Displaying this dictionary:
print(dictionary2)
Results in each key being associated with the value 1.
{'x': 1, 'y': 1, 'z': 1}
These examples succinctly demonstrate the fromkeys method's effectiveness.
A Word of Caution with Mutable Values:
When a mutable object, like a list, serves as the value, every key refers to the same object instance.
Changing the list under one key inadvertently changes it for all.
For instance, consider a dictionary with list-based keys.
keys = ['alpha', 'beta']
Assign an empty list [ ] as the common value.
value = []
Create the dictionary with fromkeys, using the keys and value.
dictionary = dict.fromkeys(keys, value)
Modifying the list for the "alpha" key:
dictionary['alpha'].append(1)
Changes the list, now containing the element 1.
Observe the dictionary now:
print(dictionary)
Both keys reflect the updated list, illustrating shared references.
{'alpha': [1], 'beta': [1]}
In summary, Python's fromkeys is invaluable for efficiently creating dictionaries with preset values. Its correct application, particularly with mutable values, is essential to prevent unintended behavior.